Introduction
FormKit’s Drag and Drop is a small library for adding data-first drag and drop sorting and transferring of elements in your app. It’s simple, flexible, framework agnostic, and clocks in at only ~4Kb gzipped. Drag and Drop works with React, Solid, Vue, Svelte, or any JavaScript application.
Getting Started
Install
Drag and Drop is published as @formkit/drag-and-drop
on npm. Click to copy the install command for your package manager of choice:
- npm install @formkit/drag-and-drop
- pnpm add @formkit/drag-and-drop
- yarn add @formkit/drag-and-drop
- bun install @formkit/drag-and-drop
Usage
Drag and drop ships two main functions: dragAndDrop
and useDragAndDrop
. These can be imported for your framework of choice by using the subpath import @formkit/drag-and-drop/react
. A native JavaScript version of the dragAndDrop
function is also available at @formkit/drag-and-drop
.
useDragAndDrop
The useDragAndDrop
hook is the most convenient way to add Drag and Drop to your app. Call this function with an initial array of values. It returns a tuple containing a a template ref and a reactive array of values. The template ref should be assigned to the parent DOM element of the items you wish to make draggable. The reactive array of values should be used in your template to render the draggable elements.
// React:const [parentRef, values, setValues] = useDragAndDrop(
['Item 1', 'Item2', 'Item3']
)
// Vue:const [parentRef, values] = useDragAndDrop(
['Item 1', 'Item2', 'Item3']
)
dragAndDrop
The dragAndDrop
hook allows you to define your own ref and list state. This is useful if you need to integrate with a pre-existing app without changing your component structure.
// React:dragAndDrop({
parent: parentRef,
state: [values, setValues]
})
// Vue:dragAndDrop({
parent: parentRef,
value: valueRef
})
Features
Drag and drop ships out of the box with the ability to sort and transfer individual items between lists. Further customization is also available via first-party plugins, and of course you can always write your own.
Sortability
Using useDragAndDrop
or dragAndDrop
automatically makes a lists sortable. Dragging items within your list will automatically change your list’s state to reflect the new order (which in turn allows the framework to re-render to the correct order).
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
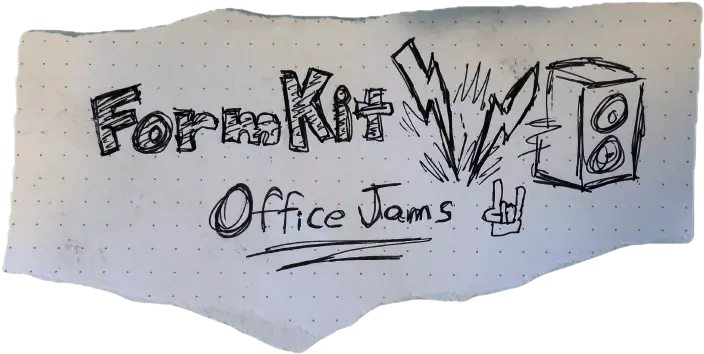
- Depeche Mode
- Duran Duran
- Pet Shop Boys
- Kraftwerk
- Tears for Fears
- Spandau Ballet
If there is a direct descendant of the parent that should not become draggable, set the draggable
property of the parent config. draggable
is a callback function that lets you determine whether or not a given element should be draggable.
Draggable
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.id?: string | undefined
id="no-drag">I am NOT draggable</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.id?: string | undefined
id="no-drag">I am NOT draggable</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
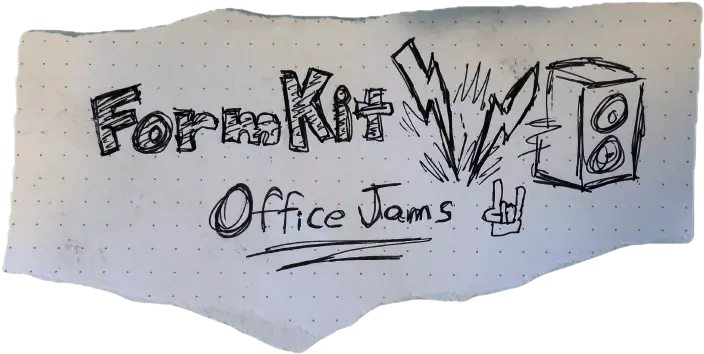
- Depeche Mode
- Duran Duran
- Pet Shop Boys
- Kraftwerk
- Tears for Fears
- Spandau Ballet
- I am NOT draggable
Transferability
If the intention is to transfer values between lists, simply set the group
property in the configuration to the same value for each list.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
Complete
- Pickup new mix-tape from Beth
Disable sort
If you would like to prevent sorting within a given list, but still allow transfer, set the sortable
property to false
in the configuration.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
Complete
- Pickup new mix-tape from Beth
Accepts
For a more nuanced transfer experience, define the accepts
property in the configuration. This property is a callback function that lets you determine whether or not a given element can be transferred into the given list.
- React
- Vue
- Native
import React from "react";
import type { interface ParentConfig<T>
The configuration object for a given parent.ParentConfig } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export default function function myComponent(): React.JSX.Element
myComponent() {
const [const source: React.RefObject<HTMLUListElement>
source, const items1: string[]
items1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["dungeon_master.exe", "map_1.dat", "map_2.dat", "character1.txt", "character2.txt", "shell32.dll", "README.txt",],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
const const config1: Partial<ParentConfig<string>>
config1: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config1: Partial<ParentConfig<string>>
config1.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, lastParentData: ParentRecord<string>, state: DragState<...> | TouchState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target2: React.RefObject<HTMLElement>
target2.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items2: string[]
items2.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 3;
};
const const config2: Partial<ParentConfig<string>>
config2: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config2: Partial<ParentConfig<string>>
config2.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, lastParentData: ParentRecord<string>, state: DragState<...> | TouchState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target1: React.RefObject<HTMLElement>
target1.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items3: string[]
items3.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 5;
};
const [const target1: React.RefObject<HTMLElement>
target1, const items2: string[]
items2] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["knight.bmp", "dragon.bmp"], const config1: Partial<ParentConfig<string>>
config1);
const [const target2: React.RefObject<HTMLElement>
target2, const items3: string[]
items3] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["brick.bmp", "moss.bmp"], const config2: Partial<ParentConfig<string>>
config2);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const source: React.RefObject<HTMLUListElement>
source}>
{const items1: string[]
items1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target1">
{const items2: string[]
items2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target2">
{const items3: string[]
items3.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import type { interface ParentConfig<T>
The configuration object for a given parent.ParentConfig } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export default function function myComponent(): React.JSX.Element
myComponent() {
const [const source: React.RefObject<HTMLUListElement>
source, const items1: string[]
items1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["dungeon_master.exe", "map_1.dat", "map_2.dat", "character1.txt", "character2.txt", "shell32.dll", "README.txt",],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
const const config1: Partial<ParentConfig<string>>
config1: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config1: Partial<ParentConfig<string>>
config1.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, lastParentData: ParentRecord<string>, state: DragState<...> | TouchState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target2: React.RefObject<HTMLElement>
target2.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items2: string[]
items2.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 3;
};
const const config2: Partial<ParentConfig<string>>
config2: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config2: Partial<ParentConfig<string>>
config2.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, lastParentData: ParentRecord<string>, state: DragState<...> | TouchState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target1: React.RefObject<HTMLElement>
target1.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items3: string[]
items3.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 5;
};
const [const target1: React.RefObject<HTMLElement>
target1, const items2: string[]
items2] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["knight.bmp", "dragon.bmp"], const config1: Partial<ParentConfig<string>>
config1);
const [const target2: React.RefObject<HTMLElement>
target2, const items3: string[]
items3] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["brick.bmp", "moss.bmp"], const config2: Partial<ParentConfig<string>>
config2);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const source: React.RefObject<HTMLUListElement>
source}>
{const items1: string[]
items1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target1">
{const items2: string[]
items2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target2">
{const items3: string[]
items3.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
- dungeon_master.exe
- map_1.dat
- map_2.dat
- character1.txt
- character2.txt
- shell32.dll
- README.txt
- knight.bmp
- dragon.bmp
- brick.bmp
- moss.bmp
Drag Handles
Drag handles are a common way to allow users to drag items without accidentally dragging them when they don't intend to. To implement drag handles, set the dragHandle
property to a selector that will be used to find the handle.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth", "Implement drag handles"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the node.dragHandle: ".kanban-handle"
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the node.dragHandle: ".kanban-handle"
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth", "Implement drag handles"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the node.dragHandle: ".kanban-handle"
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the node.dragHandle: ".kanban-handle"
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
Complete
- Pickup new mix-tape from Beth
- Implement drag handles
Updating config
The parent config can be updated at any time by using the updateConfig
method returned from useDragAndDrop
. Alternatively, the parent config can be updated by importing updateConfig
from @formkit/drag-and-drop
.
- React
- Vue
- Native
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, Dispatch<SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const values: string[]
values, const _setValues: React.Dispatch<React.SetStateAction<string[]>>
_setValues, const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<
HTMLUListElement,
string
>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
const [const disabled: boolean
disabled, const setDisabled: React.Dispatch<React.SetStateAction<boolean>>
setDisabled] = useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const toggleDisabled: () => void
toggleDisabled = () => {
const setDisabled: (value: React.SetStateAction<boolean>) => void
setDisabled(!const disabled: boolean
disabled);
const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig({ disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled: !const disabled: boolean
disabled });
};
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const values: string[]
values.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={const toggleDisabled: () => void
toggleDisabled}>
{const disabled: boolean
disabled ? "Enable" : "Disable"} drag and drop
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, Dispatch<SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const values: string[]
values, const _setValues: React.Dispatch<React.SetStateAction<string[]>>
_setValues, const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<
HTMLUListElement,
string
>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
const [const disabled: boolean
disabled, const setDisabled: React.Dispatch<React.SetStateAction<boolean>>
setDisabled] = useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const toggleDisabled: () => void
toggleDisabled = () => {
const setDisabled: (value: React.SetStateAction<boolean>) => void
setDisabled(!const disabled: boolean
disabled);
const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig({ disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled: !const disabled: boolean
disabled });
};
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const values: string[]
values.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={const toggleDisabled: () => void
toggleDisabled}>
{const disabled: boolean
disabled ? "Enable" : "Disable"} drag and drop
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
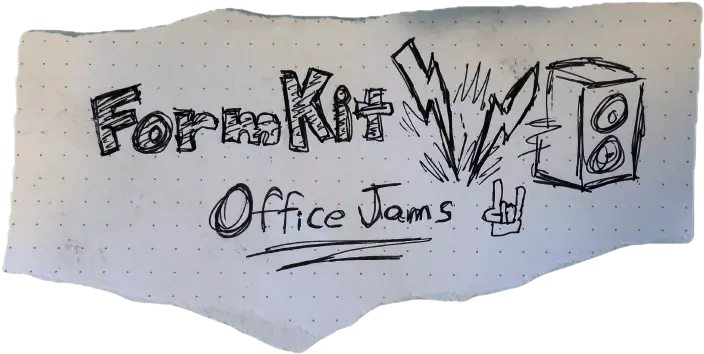
- Depeche Mode
- Duran Duran
- Pet
- Kraftwerk
- Tears for Fears
- Spandau Ballet
Plugins
Plugins are a powerful way to extend the functionality of the library. They can be used to add new features, modify existing ones, or even create entirely new experiences.
Animations
To add animations to your drag and drop experience, you can use the first-party animations
plugin.
- React
- Vue
- Native
import React from "react";
import { function animations(animationsConfig?: AnimationsConfig): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{ plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [function animations(animationsConfig?: AnimationsConfig | undefined): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations()] }
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
import React from "react";
import { function animations(animationsConfig?: AnimationsConfig): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{ plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [function animations(animationsConfig?: AnimationsConfig | undefined): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations()] }
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
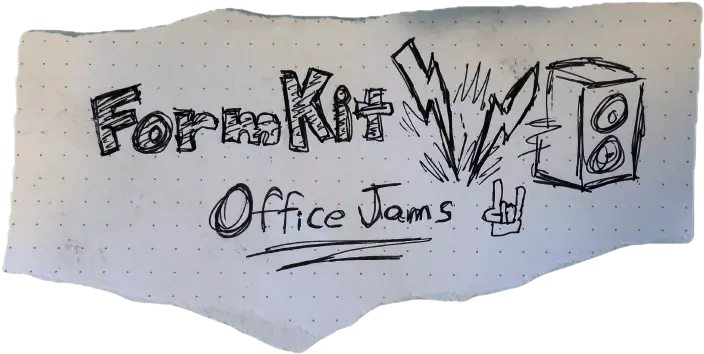
- Depeche Mode
- Duran Duran
- Pet Shop Boys
- Kraftwerk
- Tears for Fears
- Spandau Ballet
Multi Drag
The multi-drag plugin allows you to select multiple items and drag them together.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
import { function multiDrag<T>(multiDragConfig?: Partial<MultiDragConfig<T>>): (parent: HTMLElement) => {
setup(): void;
tearDownNodeRemap<T>(data: TearDownNodeData<T>): void;
tearDownNode<T_1>(data: TearDownNodeData<T_1>): void;
setupNodeRemap<T_2>(data: SetupNodeData<...>): void;
setupNode<T_3>(data: SetupNodeData<...>): void;
} | undefined
multiDrag, function selections<T>(selectionsConfig?: SelectionsConfig<T>): (parent: HTMLElement) => {
setup(): void;
tearDown(): void;
tearDownNode<T>(data: TearDownNodeData<T>): void;
setupNode<T_1>(data: SetupNodeData<T_1>): void;
} | undefined
selections } from "@formkit/drag-and-drop";
export function function myComponent(): React.JSX.Element
myComponent() {
const const mockFileNames: string[]
mockFileNames = ["dungeon_master.exe", "map_1.dat", "map_2.dat", "character1.txt", "character2.txt", "shell32.dll", "README.txt"];
const [const parent1: React.RefObject<HTMLUListElement>
parent1, const files1: string[]
files1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const mockFileNames: string[]
mockFileNames,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
multiDrag<unknown>(multiDragConfig?: Partial<MultiDragConfig<unknown>> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDownNodeRemap<T>(data: TearDownNodeData<T>): void;
tearDownNode<T_1>(data: TearDownNodeData<...>): void;
setupNodeRemap<T_2>(data: SetupNodeData<...>): void;
setupNode<T_3>(data: SetupNodeData<...>): void;
} | undefined
multiDrag({
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
selections<unknown>(selectionsConfig?: SelectionsConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDown(): void;
tearDownNode<T>(data: TearDownNodeData<T>): void;
setupNode<T_1>(data: SetupNodeData<...>): void;
} | undefined
selections({
SelectionsConfig<T>.selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
}),
],
}),
],
}
);
const [const parent2: React.RefObject<HTMLUListElement>
parent2, const files2: string[]
files2] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([], {
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
multiDrag<unknown>(multiDragConfig?: Partial<MultiDragConfig<unknown>> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDownNodeRemap<T>(data: TearDownNodeData<T>): void;
tearDownNode<T_1>(data: TearDownNodeData<...>): void;
setupNodeRemap<T_2>(data: SetupNodeData<...>): void;
setupNode<T_3>(data: SetupNodeData<...>): void;
} | undefined
multiDrag({
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
selections<unknown>(selectionsConfig?: SelectionsConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDown(): void;
tearDownNode<T>(data: TearDownNodeData<T>): void;
setupNode<T_1>(data: SetupNodeData<...>): void;
} | undefined
selections({
SelectionsConfig<T>.selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
}),
],
}),
],
});
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="file-manager">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent1: React.RefObject<HTMLUListElement>
parent1} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files1: string[]
files1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">{file: string
file}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent2: React.RefObject<HTMLUListElement>
parent2} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files2: string[]
files2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">{file: string
file}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
import { function multiDrag<T>(multiDragConfig?: Partial<MultiDragConfig<T>>): (parent: HTMLElement) => {
setup(): void;
tearDownNodeRemap<T>(data: TearDownNodeData<T>): void;
tearDownNode<T_1>(data: TearDownNodeData<T_1>): void;
setupNodeRemap<T_2>(data: SetupNodeData<...>): void;
setupNode<T_3>(data: SetupNodeData<...>): void;
} | undefined
multiDrag, function selections<T>(selectionsConfig?: SelectionsConfig<T>): (parent: HTMLElement) => {
setup(): void;
tearDown(): void;
tearDownNode<T>(data: TearDownNodeData<T>): void;
setupNode<T_1>(data: SetupNodeData<T_1>): void;
} | undefined
selections } from "@formkit/drag-and-drop";
export function function myComponent(): React.JSX.Element
myComponent() {
const const mockFileNames: string[]
mockFileNames = ["dungeon_master.exe", "map_1.dat", "map_2.dat", "character1.txt", "character2.txt", "shell32.dll", "README.txt"];
const [const parent1: React.RefObject<HTMLUListElement>
parent1, const files1: string[]
files1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const mockFileNames: string[]
mockFileNames,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
multiDrag<unknown>(multiDragConfig?: Partial<MultiDragConfig<unknown>> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDownNodeRemap<T>(data: TearDownNodeData<T>): void;
tearDownNode<T_1>(data: TearDownNodeData<...>): void;
setupNodeRemap<T_2>(data: SetupNodeData<...>): void;
setupNode<T_3>(data: SetupNodeData<...>): void;
} | undefined
multiDrag({
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
selections<unknown>(selectionsConfig?: SelectionsConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDown(): void;
tearDownNode<T>(data: TearDownNodeData<T>): void;
setupNode<T_1>(data: SetupNodeData<...>): void;
} | undefined
selections({
SelectionsConfig<T>.selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
}),
],
}),
],
}
);
const [const parent2: React.RefObject<HTMLUListElement>
parent2, const files2: string[]
files2] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([], {
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
multiDrag<unknown>(multiDragConfig?: Partial<MultiDragConfig<unknown>> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDownNodeRemap<T>(data: TearDownNodeData<T>): void;
tearDownNode<T_1>(data: TearDownNodeData<...>): void;
setupNodeRemap<T_2>(data: SetupNodeData<...>): void;
setupNode<T_3>(data: SetupNodeData<...>): void;
} | undefined
multiDrag({
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
selections<unknown>(selectionsConfig?: SelectionsConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
tearDown(): void;
tearDownNode<T>(data: TearDownNodeData<T>): void;
setupNode<T_1>(data: SetupNodeData<...>): void;
} | undefined
selections({
SelectionsConfig<T>.selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
}),
],
}),
],
});
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="file-manager">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent1: React.RefObject<HTMLUListElement>
parent1} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files1: string[]
files1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">{file: string
file}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent2: React.RefObject<HTMLUListElement>
parent2} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files2: string[]
files2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">{file: string
file}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
- dungeon_master.exe
- map_1.dat
- map_2.dat
- character1.txt
- character2.txt
- shell32.dll
- README.txt
Notice in the last example we are passing a separate plugin to the multi-drag plugin. This is the selections plugin, which sets things like click handlers. If you would like to write your own logic (or have already written logic for selecting items), you can pass your own selected values to the selections
property.
Custom Plugins
You can create your own plugins to extend the functionality of the library. Here is an example of a custom plugin that adds a drag and drop feature to a list of flavors.
Plugins accept a parent element and return an object with methods that are called internally at different lifecycles:
setup
andtearDown
are called whenuseDragAndDrop
ordragAndDrop
is called.setupNode
andtearDownNode
are also called when parent is initialized and accept data representing a given node (or child).setupNodeRemap
andtearDownNodeRemap
are called when the value of the parent is changed and the nodes are remapped.
- React
- Vue
- Native
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, Dispatch<SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import type { type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
import { const parents: ParentsData<any>
parents, function addEvents(el: Document | ShadowRoot | Node | HTMLElement, events: EventHandlers | any): AbortController
Takes a given el and event handlers, assigns them, and returns the used abort
controller.addEvents } from "@formkit/drag-and-drop";
function function App(): React.JSX.Element
App() {
const [const dragStatus: string
dragStatus, const setDragStatus: React.Dispatch<React.SetStateAction<string>>
setDragStatus] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not dragging");
const [const dragCount: number
dragCount, const setDragCount: React.Dispatch<React.SetStateAction<number>>
setDragCount] = useState<number>(initialState: number | (() => number)): [number, React.Dispatch<React.SetStateAction<number>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(0);
const const dragStatusPlugin: DNDPlugin
dragStatusPlugin: type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin = (parent: HTMLElement
parent) => {
const const parentData: ParentData<any> | undefined
parentData = const parents: ParentsData<any>
parents.WeakMap<HTMLElement, ParentData<any>>.get(key: HTMLElement): ParentData<any> | undefined
get(parent: HTMLElement
parent);
if (!const parentData: ParentData<any> | undefined
parentData) return;
function function (local function) dragstart(event: DragEvent): void
dragstart(event: DragEvent
event: DragEvent) {
const const node: HTMLElement
node = event: DragEvent
event.Event.target: EventTarget | null
Returns the object to which event is dispatched (its target).
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Event/target)target as HTMLElement;
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus(`Dragging ${const node: HTMLElement
node.Node.textContent: string | null
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Node/textContent)textContent}`);
const setDragCount: (value: React.SetStateAction<number>) => void
setDragCount((count: number
count) => count: number
count + 1);
}
function function (local function) dragend(): void
dragend() {
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Not dragging");
}
return {
DNDPluginData.setup?: (() => void) | undefined
The function to call when the parent is set up.setup() {},
function teardown(): void
teardown() {},
DNDPluginData.setupNode?: SetupNode | undefined
Called when entry point function is invoked on parent.setupNode(data: SetupNodeData<T>
data) {
data: SetupNodeData<T>
data.SetupNodeData<T>.nodeData: NodeData<T>
The data of the node that is being set up.nodeData.NodeData<T>.abortControllers: Record<string, AbortController>
The abort controllers for the node.abortControllers.AbortController
A controller object that allows you to abort one or more DOM requests as and when desired.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/AbortController)customPlugin = function addEvents(el: HTMLElement | Document | ShadowRoot | Node, events: any): AbortController
Takes a given el and event handlers, assigns them, and returns the used abort
controller.addEvents(data: SetupNodeData<T>
data.SetupNodeData<T>.node: Node
The node that is being set up.node, {
dragstart: (event: DragEvent) => void
dragstart: function (local function) dragstart(event: DragEvent): void
dragstart,
dragend: () => void
dragend: function (local function) dragend(): void
dragend,
});
},
DNDPluginData.tearDownNode?: TearDownNode | undefined
Called when entry point function is invoked on parent.tearDownNode(data: TearDownNodeData<T>
data) {
if (data: TearDownNodeData<T>
data.TearDownNodeData<T>.nodeData?: NodeData<T> | undefined
The data of the node that is being torn down.nodeData?.NodeData<T>.abortControllers: Record<string, AbortController> | undefined
The abort controllers for the node.abortControllers?.AbortController | undefined
customPlugin) {
data: TearDownNodeData<T>
data.TearDownNodeData<T>.nodeData?: NodeData<T>
The data of the node that is being torn down.nodeData?.NodeData<T>.abortControllers: Record<string, AbortController>
The abort controllers for the node.abortControllers?.AbortController
A controller object that allows you to abort one or more DOM requests as and when desired.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/AbortController)customPlugin.AbortController.abort(reason?: any): void
Invoking this method will set this object's AbortSignal's aborted flag and signal to any observers that the associated activity is to be aborted.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/AbortController/abort)abort();
}
},
DNDPluginData.setupNodeRemap?: SetupNode | undefined
Called anytime the nodes are mutatedsetupNodeRemap() {},
DNDPluginData.tearDownNodeRemap?: TearDownNode | undefined
Called when the parent is dragged over.tearDownNodeRemap() {},
};
};
const [const parent: React.RefObject<HTMLUListElement>
parent, const items: string[]
items] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["🍦 vanilla", "🍫 chocolate", "🍓 strawberry"],
{ plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [const dragStatusPlugin: DNDPlugin
dragStatusPlugin] }
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>Rank your favorite flavors</JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragStatus: string
dragStatus}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragCount: number
dragCount}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const items: string[]
items.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => {
return <JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>;
})}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
export default function App(): React.JSX.Element
App;
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, Dispatch<SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import type { type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [RefObject<E>, T[], Dispatch<SetStateAction<T[]>>, (config: Partial<ParentConfig<T>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
import { const parents: ParentsData<any>
parents, function addEvents(el: Document | ShadowRoot | Node | HTMLElement, events: EventHandlers | any): AbortController
Takes a given el and event handlers, assigns them, and returns the used abort
controller.addEvents } from "@formkit/drag-and-drop";
function function App(): React.JSX.Element
App() {
const [const dragStatus: string
dragStatus, const setDragStatus: React.Dispatch<React.SetStateAction<string>>
setDragStatus] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not dragging");
const [const dragCount: number
dragCount, const setDragCount: React.Dispatch<React.SetStateAction<number>>
setDragCount] = useState<number>(initialState: number | (() => number)): [number, React.Dispatch<React.SetStateAction<number>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(0);
const const dragStatusPlugin: DNDPlugin
dragStatusPlugin: type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin = (parent: HTMLElement
parent) => {
const const parentData: ParentData<any> | undefined
parentData = const parents: ParentsData<any>
parents.WeakMap<HTMLElement, ParentData<any>>.get(key: HTMLElement): ParentData<any> | undefined
get(parent: HTMLElement
parent);
if (!const parentData: ParentData<any> | undefined
parentData) return;
function function (local function) dragstart(event: DragEvent): void
dragstart(event: DragEvent
event: DragEvent) {
const const node: HTMLElement
node = event: DragEvent
event.Event.target: EventTarget | null
Returns the object to which event is dispatched (its target).
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Event/target)target as HTMLElement;
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus(`Dragging ${const node: HTMLElement
node.Node.textContent: string | null
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Node/textContent)textContent}`);
const setDragCount: (value: React.SetStateAction<number>) => void
setDragCount((count: number
count) => count: number
count + 1);
}
function function (local function) dragend(): void
dragend() {
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Not dragging");
}
return {
DNDPluginData.setup?: (() => void) | undefined
The function to call when the parent is set up.setup() {},
function teardown(): void
teardown() {},
DNDPluginData.setupNode?: SetupNode | undefined
Called when entry point function is invoked on parent.setupNode(data: SetupNodeData<T>
data) {
data: SetupNodeData<T>
data.SetupNodeData<T>.nodeData: NodeData<T>
The data of the node that is being set up.nodeData.NodeData<T>.abortControllers: Record<string, AbortController>
The abort controllers for the node.abortControllers.AbortController
A controller object that allows you to abort one or more DOM requests as and when desired.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/AbortController)customPlugin = function addEvents(el: HTMLElement | Document | ShadowRoot | Node, events: any): AbortController
Takes a given el and event handlers, assigns them, and returns the used abort
controller.addEvents(data: SetupNodeData<T>
data.SetupNodeData<T>.node: Node
The node that is being set up.node, {
dragstart: (event: DragEvent) => void
dragstart: function (local function) dragstart(event: DragEvent): void
dragstart,
dragend: () => void
dragend: function (local function) dragend(): void
dragend,
});
},
DNDPluginData.tearDownNode?: TearDownNode | undefined
Called when entry point function is invoked on parent.tearDownNode(data: TearDownNodeData<T>
data) {
if (data: TearDownNodeData<T>
data.TearDownNodeData<T>.nodeData?: NodeData<T> | undefined
The data of the node that is being torn down.nodeData?.NodeData<T>.abortControllers: Record<string, AbortController> | undefined
The abort controllers for the node.abortControllers?.AbortController | undefined
customPlugin) {
data: TearDownNodeData<T>
data.TearDownNodeData<T>.nodeData?: NodeData<T>
The data of the node that is being torn down.nodeData?.NodeData<T>.abortControllers: Record<string, AbortController>
The abort controllers for the node.abortControllers?.AbortController
A controller object that allows you to abort one or more DOM requests as and when desired.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/AbortController)customPlugin.AbortController.abort(reason?: any): void
Invoking this method will set this object's AbortSignal's aborted flag and signal to any observers that the associated activity is to be aborted.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/AbortController/abort)abort();
}
},
DNDPluginData.setupNodeRemap?: SetupNode | undefined
Called anytime the nodes are mutatedsetupNodeRemap() {},
DNDPluginData.tearDownNodeRemap?: TearDownNode | undefined
Called when the parent is dragged over.tearDownNodeRemap() {},
};
};
const [const parent: React.RefObject<HTMLUListElement>
parent, const items: string[]
items] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["🍦 vanilla", "🍫 chocolate", "🍓 strawberry"],
{ plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [const dragStatusPlugin: DNDPlugin
dragStatusPlugin] }
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>Rank your favorite flavors</JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragStatus: string
dragStatus}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragCount: number
dragCount}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.ClassAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const items: string[]
items.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => {
return <JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>;
})}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
export default function App(): React.JSX.Element
App;
Configuration
Core
Each list can be passed a configuration object. This object can be used to set options like group
, drag handles
, and or more dynamic options such as determining which direct descendants of a list are draggable
. Invocation of useDragAndDrop
or dragAndDrop
can be used idempotently to update the configuration for a given list.
import type {
interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord,
interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState,
interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState,
interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData,
interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData,
interface NodeEventData<T>
The data passed to the node event listener.NodeEventData,
type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin,
interface TouchOverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.TouchOverNodeEvent,
interface TouchOverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.TouchOverParentEvent,
type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode,
interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData,
type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode,
} from "@formkit/drag-and-drop";
/**
* The configuration object for a given parent.
*/
export interface interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<function (type parameter) T in ParentConfig<T>
T> {
[key: string
key: string]: any;
/**
* A function that returns whether a given parent accepts a given node.
*/
ParentConfig<T>.accepts?: ((targetParentData: ParentRecord<T>, initialParentData: ParentRecord<T>, lastParentData: ParentRecord<T>, state: DragState<T> | TouchState<T>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts?: (
targetParentData: ParentRecord<T>
targetParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
initialParentData: ParentRecord<T>
initialParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
lastParentData: ParentRecord<T>
lastParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T> | TouchState<T>
state: interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState<function (type parameter) T in ParentConfig<T>
T> | interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState<function (type parameter) T in ParentConfig<T>
T>
) => boolean;
/**
* A flag to disable dragability of all nodes in the parent.
*/
ParentConfig<T>.disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled?: boolean;
/**
* A selector for the drag handle. Will search at any depth within the node.
*/
ParentConfig<T>.dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the node.dragHandle?: string;
/**
* A function that returns whether a given node is draggable.
*/
ParentConfig<T>.draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable?: (child: HTMLElement
child: HTMLElement) => boolean;
/**
* The class to add to a node when it is being dragged.
*/
ParentConfig<T>.draggingClass?: string | undefined
The class to add to a node when it is being dragged.draggingClass?: string;
/**
* The class to add to a node when the node is dragged over it.
*/
ParentConfig<T>.dropZoneClass?: string | undefined
The class to add to a node when the node is dragged over it.dropZoneClass?: string;
/**
* A flag to indicate whether the parent itself is a dropZone.
*/
ParentConfig<T>.dropZone?: boolean | undefined
A flag to indicate whether the parent itself is a dropZone.dropZone?: boolean;
/**
* The group that the parent belongs to. This is used for allowing multiple
* parents to transfer nodes between each other.
*/
ParentConfig<T>.group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group?: string;
/**
* Function that is called when dragend or touchend event occurs.
*/
ParentConfig<T>.handleEnd: (data: NodeDragEventData<T> | NodeTouchEventData<T>) => void
Function that is called when dragend or touchend event occurs.handleEnd: (data: NodeDragEventData<T> | NodeTouchEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T> | interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when dragstart event occurs.
*/
ParentConfig<T>.handleDragstart: (data: NodeDragEventData<T>) => void
Function that is called when dragstart event occurs.handleDragstart: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when touchstart event occurs.
*/
ParentConfig<T>.handleTouchstart: (data: NodeTouchEventData<T>) => void
Function that is called when touchstart event occurs.handleTouchstart: (data: NodeTouchEventData<T>
data: interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a dragover event is triggered on the parent.
*/
ParentConfig<T>.handleDragoverParent: (data: ParentEventData<T>) => void
Function that is called when a dragover event is triggered on the parent.handleDragoverParent: (data: ParentEventData<T>
data: interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a dragover event is triggered on a node.
*/
ParentConfig<T>.handleDragoverNode: (data: NodeDragEventData<T>) => void
Function that is called when a dragover event is triggered on a node.handleDragoverNode: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a touchmove event is triggered on a node.
*/
ParentConfig<T>.handleTouchmove: (data: NodeTouchEventData<T>) => void
Function that is called when a touchmove event is triggered on a node.handleTouchmove: (data: NodeTouchEventData<T>
data: interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over a given node (similar to dragover).
*/
ParentConfig<T>.handleTouchOverNode: (data: TouchOverNodeEvent<T>) => void
Function that is called when a node that is being moved by touchmove event
is over a given node (similar to dragover).handleTouchOverNode: (data: TouchOverNodeEvent<T>
data: interface TouchOverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.TouchOverNodeEvent<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over the parent (similar to dragover).
*/
ParentConfig<T>.handleTouchOverParent: (e: TouchOverParentEvent<T>) => void
Function that is called when a node that is being moved by touchmove event
is over the parent (similar to dragover).handleTouchOverParent: (e: TouchOverParentEvent<T>
e: interface TouchOverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.TouchOverParentEvent<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* A flag to indicate whether long touch is enabled.
*/
ParentConfig<T>.longTouch?: boolean | undefined
A flag to indicate whether long touch is enabled.longTouch?: boolean;
/**
* The class to add to a node when a long touch action is performed.
*/
ParentConfig<T>.longTouchClass?: string | undefined
The class to add to a node when a long touch action is performed.longTouchClass?: string;
/**
* The time in milliseconds to wait before a long touch is performed.
*/
ParentConfig<T>.longTouchTimeout?: number | undefined
The time in milliseconds to wait before a long touch is performed.longTouchTimeout?: number;
/**
* The name of the parent (used for accepts function for increased specificity).
*/
ParentConfig<T>.name?: string | undefined
The name of the parent (used for accepts function for increased specificity).name?: string;
/**
* Function that is called when a sort operation is to be performed.
*/
ParentConfig<T>.performSort: (state: DragState<T> | TouchState<T>, data: NodeDragEventData<T> | NodeTouchEventData<T>) => void
Function that is called when a sort operation is to be performed.performSort: (
state: DragState<T> | TouchState<T>
state: interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState<function (type parameter) T in ParentConfig<T>
T> | interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState<function (type parameter) T in ParentConfig<T>
T>,
data: NodeDragEventData<T> | NodeTouchEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T> | interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a transfer operation is to be performed.
*/
ParentConfig<T>.performTransfer: (state: DragState<T> | TouchState<T>, data: NodeEventData<T> | ParentEventData<T>) => void
Function that is called when a transfer operation is to be performed.performTransfer: (
state: DragState<T> | TouchState<T>
state: interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState<function (type parameter) T in ParentConfig<T>
T> | interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState<function (type parameter) T in ParentConfig<T>
T>,
data: ParentEventData<T> | NodeEventData<T>
data: interface NodeEventData<T>
The data passed to the node event listener.NodeEventData<function (type parameter) T in ParentConfig<T>
T> | interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* An array of functions to use for a given parent.
*/
ParentConfig<T>.plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins?: interface Array<T>
Array<type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin>;
/**
* The root element to use for the parent.
*/
ParentConfig<T>.root: Document | ShadowRoot
The root element to use for the parent.root: Document | ShadowRoot;
/**
* Function that is called when a node is set up.
*/
ParentConfig<T>.setupNode: SetupNode
Function that is called when a node is set up.setupNode: type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode;
/**
* Flag for whether or not to allow sorting within a given parent.
*/
ParentConfig<T>.sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable?: boolean;
/**
* Function that is called when a node is torn down.
*/
ParentConfig<T>.tearDownNode: TearDownNode
Function that is called when a node is torn down.tearDownNode: type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode;
/**
* The threshold for a drag to be considered a valid sort
* operation.
*/
ParentConfig<T>.threshold: {
horizontal: number;
vertical: number;
}
The threshold for a drag to be considered a valid sort
operation.threshold: {
horizontal: number
horizontal: number;
vertical: number
vertical: number;
};
/**
* The class to add to a node when it is being dragged via touch.
*/
ParentConfig<T>.touchDraggingClass?: string | undefined
The class to add to a node when it is being dragged via touch.touchDraggingClass?: string;
ParentConfig<T>.touchDropZoneClass?: string | undefined
touchDropZoneClass?: string;
}
import type {
interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord,
interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState,
interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState,
interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData,
interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData,
interface NodeEventData<T>
The data passed to the node event listener.NodeEventData,
type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin,
interface TouchOverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.TouchOverNodeEvent,
interface TouchOverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.TouchOverParentEvent,
type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode,
interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData,
type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode,
} from "@formkit/drag-and-drop";
/**
* The configuration object for a given parent.
*/
export interface interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<function (type parameter) T in ParentConfig<T>
T> {
[key: string
key: string]: any;
/**
* A function that returns whether a given parent accepts a given node.
*/
ParentConfig<T>.accepts?: ((targetParentData: ParentRecord<T>, initialParentData: ParentRecord<T>, lastParentData: ParentRecord<T>, state: DragState<T> | TouchState<T>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts?: (
targetParentData: ParentRecord<T>
targetParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
initialParentData: ParentRecord<T>
initialParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
lastParentData: ParentRecord<T>
lastParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T> | TouchState<T>
state: interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState<function (type parameter) T in ParentConfig<T>
T> | interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState<function (type parameter) T in ParentConfig<T>
T>
) => boolean;
/**
* A flag to disable dragability of all nodes in the parent.
*/
ParentConfig<T>.disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled?: boolean;
/**
* A selector for the drag handle. Will search at any depth within the node.
*/
ParentConfig<T>.dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the node.dragHandle?: string;
/**
* A function that returns whether a given node is draggable.
*/
ParentConfig<T>.draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable?: (child: HTMLElement
child: HTMLElement) => boolean;
/**
* The class to add to a node when it is being dragged.
*/
ParentConfig<T>.draggingClass?: string | undefined
The class to add to a node when it is being dragged.draggingClass?: string;
/**
* The class to add to a node when the node is dragged over it.
*/
ParentConfig<T>.dropZoneClass?: string | undefined
The class to add to a node when the node is dragged over it.dropZoneClass?: string;
/**
* A flag to indicate whether the parent itself is a dropZone.
*/
ParentConfig<T>.dropZone?: boolean | undefined
A flag to indicate whether the parent itself is a dropZone.dropZone?: boolean;
/**
* The group that the parent belongs to. This is used for allowing multiple
* parents to transfer nodes between each other.
*/
ParentConfig<T>.group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group?: string;
/**
* Function that is called when dragend or touchend event occurs.
*/
ParentConfig<T>.handleEnd: (data: NodeDragEventData<T> | NodeTouchEventData<T>) => void
Function that is called when dragend or touchend event occurs.handleEnd: (data: NodeDragEventData<T> | NodeTouchEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T> | interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when dragstart event occurs.
*/
ParentConfig<T>.handleDragstart: (data: NodeDragEventData<T>) => void
Function that is called when dragstart event occurs.handleDragstart: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when touchstart event occurs.
*/
ParentConfig<T>.handleTouchstart: (data: NodeTouchEventData<T>) => void
Function that is called when touchstart event occurs.handleTouchstart: (data: NodeTouchEventData<T>
data: interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a dragover event is triggered on the parent.
*/
ParentConfig<T>.handleDragoverParent: (data: ParentEventData<T>) => void
Function that is called when a dragover event is triggered on the parent.handleDragoverParent: (data: ParentEventData<T>
data: interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a dragover event is triggered on a node.
*/
ParentConfig<T>.handleDragoverNode: (data: NodeDragEventData<T>) => void
Function that is called when a dragover event is triggered on a node.handleDragoverNode: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a touchmove event is triggered on a node.
*/
ParentConfig<T>.handleTouchmove: (data: NodeTouchEventData<T>) => void
Function that is called when a touchmove event is triggered on a node.handleTouchmove: (data: NodeTouchEventData<T>
data: interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over a given node (similar to dragover).
*/
ParentConfig<T>.handleTouchOverNode: (data: TouchOverNodeEvent<T>) => void
Function that is called when a node that is being moved by touchmove event
is over a given node (similar to dragover).handleTouchOverNode: (data: TouchOverNodeEvent<T>
data: interface TouchOverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.TouchOverNodeEvent<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over the parent (similar to dragover).
*/
ParentConfig<T>.handleTouchOverParent: (e: TouchOverParentEvent<T>) => void
Function that is called when a node that is being moved by touchmove event
is over the parent (similar to dragover).handleTouchOverParent: (e: TouchOverParentEvent<T>
e: interface TouchOverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.TouchOverParentEvent<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* A flag to indicate whether long touch is enabled.
*/
ParentConfig<T>.longTouch?: boolean | undefined
A flag to indicate whether long touch is enabled.longTouch?: boolean;
/**
* The class to add to a node when a long touch action is performed.
*/
ParentConfig<T>.longTouchClass?: string | undefined
The class to add to a node when a long touch action is performed.longTouchClass?: string;
/**
* The time in milliseconds to wait before a long touch is performed.
*/
ParentConfig<T>.longTouchTimeout?: number | undefined
The time in milliseconds to wait before a long touch is performed.longTouchTimeout?: number;
/**
* The name of the parent (used for accepts function for increased specificity).
*/
ParentConfig<T>.name?: string | undefined
The name of the parent (used for accepts function for increased specificity).name?: string;
/**
* Function that is called when a sort operation is to be performed.
*/
ParentConfig<T>.performSort: (state: DragState<T> | TouchState<T>, data: NodeDragEventData<T> | NodeTouchEventData<T>) => void
Function that is called when a sort operation is to be performed.performSort: (
state: DragState<T> | TouchState<T>
state: interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState<function (type parameter) T in ParentConfig<T>
T> | interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState<function (type parameter) T in ParentConfig<T>
T>,
data: NodeDragEventData<T> | NodeTouchEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T> | interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a transfer operation is to be performed.
*/
ParentConfig<T>.performTransfer: (state: DragState<T> | TouchState<T>, data: NodeEventData<T> | ParentEventData<T>) => void
Function that is called when a transfer operation is to be performed.performTransfer: (
state: DragState<T> | TouchState<T>
state: interface DragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.DragState<function (type parameter) T in ParentConfig<T>
T> | interface TouchState<T>
The state of the current drag. TouchState is only created when a touch start
event has occurred.TouchState<function (type parameter) T in ParentConfig<T>
T>,
data: ParentEventData<T> | NodeEventData<T>
data: interface NodeEventData<T>
The data passed to the node event listener.NodeEventData<function (type parameter) T in ParentConfig<T>
T> | interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* An array of functions to use for a given parent.
*/
ParentConfig<T>.plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins?: interface Array<T>
Array<type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin>;
/**
* The root element to use for the parent.
*/
ParentConfig<T>.root: Document | ShadowRoot
The root element to use for the parent.root: Document | ShadowRoot;
/**
* Function that is called when a node is set up.
*/
ParentConfig<T>.setupNode: SetupNode
Function that is called when a node is set up.setupNode: type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode;
/**
* Flag for whether or not to allow sorting within a given parent.
*/
ParentConfig<T>.sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable?: boolean;
/**
* Function that is called when a node is torn down.
*/
ParentConfig<T>.tearDownNode: TearDownNode
Function that is called when a node is torn down.tearDownNode: type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode;
/**
* The threshold for a drag to be considered a valid sort
* operation.
*/
ParentConfig<T>.threshold: {
horizontal: number;
vertical: number;
}
The threshold for a drag to be considered a valid sort
operation.threshold: {
horizontal: number
horizontal: number;
vertical: number
vertical: number;
};
/**
* The class to add to a node when it is being dragged via touch.
*/
ParentConfig<T>.touchDraggingClass?: string | undefined
The class to add to a node when it is being dragged via touch.touchDraggingClass?: string;
ParentConfig<T>.touchDropZoneClass?: string | undefined
touchDropZoneClass?: string;
}
Plugins
Multi-drag
import type {
interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData,
interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData,
type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin,
} from "@formkit/drag-and-drop";
export interface interface MultiDragConfig<T>
MultiDragConfig<function (type parameter) T in MultiDragConfig<T>
T> {
[key: string
key: string]: any;
/**
* Class added when a node is being dragged.
*/
MultiDragConfig<T>.draggingClass?: string | undefined
Class added when a node is being dragged.draggingClass?: string;
/**
* Class added when a node is being dragged over a dropZone.
*/
MultiDragConfig<T>.dropZoneClass?: string | undefined
Class added when a node is being dragged over a dropZone.dropZoneClass?: string;
/**
* Function to set which values of a given parent are "selected". This is
* called on dragstart or touchstart.
*/
MultiDragConfig<T>.selections?: ((parentValues: Array<T>, parent: HTMLElement) => Array<T>) | undefined
Function to set which values of a given parent are "selected". This is
called on dragstart or touchstart.selections?: (parentValues: T[]
parentValues: interface Array<T>
Array<function (type parameter) T in MultiDragConfig<T>
T>, parent: HTMLElement
parent: HTMLElement) => interface Array<T>
Array<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* Class added when a node is being (touch) dragged.
*/
MultiDragConfig<T>.touchDraggingClass?: string | undefined
Class added when a node is being (touch) dragged.touchDraggingClass?: string;
/**
* Class added when a node is being (touch) dragged over a dropZone.
*/
MultiDragConfig<T>.touchDropZoneClass?: string | undefined
Class added when a node is being (touch) dragged over a dropZone.touchDropZoneClass?: string;
/**
* Function that is called when dragend event occurrs event occurs.
*/
MultiDragConfig<T>.handleDragend: NodeDragEventData<T> | NodeTouchEventData<T>
Function that is called when dragend event occurrs event occurs.handleDragend: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in MultiDragConfig<T>
T> | interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* Function that is called when dragstart occurs.
*/
MultiDragConfig<T>.handleDragstart: NodeDragEventData<T>
Function that is called when dragstart occurs.handleDragstart: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* Function that is called when dragstart event occurs.
*/
MultiDragConfig<T>.handleTouchstart: NodeTouchEventData<T>
Function that is called when dragstart event occurs.handleTouchstart: interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* An array of functions to use for a given parent.
*/
MultiDragConfig<T>.plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins?: interface Array<T>
Array<type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin>;
}
import type {
interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData,
interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData,
type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin,
} from "@formkit/drag-and-drop";
export interface interface MultiDragConfig<T>
MultiDragConfig<function (type parameter) T in MultiDragConfig<T>
T> {
[key: string
key: string]: any;
/**
* Class added when a node is being dragged.
*/
MultiDragConfig<T>.draggingClass?: string | undefined
Class added when a node is being dragged.draggingClass?: string;
/**
* Class added when a node is being dragged over a dropZone.
*/
MultiDragConfig<T>.dropZoneClass?: string | undefined
Class added when a node is being dragged over a dropZone.dropZoneClass?: string;
/**
* Function to set which values of a given parent are "selected". This is
* called on dragstart or touchstart.
*/
MultiDragConfig<T>.selections?: ((parentValues: Array<T>, parent: HTMLElement) => Array<T>) | undefined
Function to set which values of a given parent are "selected". This is
called on dragstart or touchstart.selections?: (parentValues: T[]
parentValues: interface Array<T>
Array<function (type parameter) T in MultiDragConfig<T>
T>, parent: HTMLElement
parent: HTMLElement) => interface Array<T>
Array<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* Class added when a node is being (touch) dragged.
*/
MultiDragConfig<T>.touchDraggingClass?: string | undefined
Class added when a node is being (touch) dragged.touchDraggingClass?: string;
/**
* Class added when a node is being (touch) dragged over a dropZone.
*/
MultiDragConfig<T>.touchDropZoneClass?: string | undefined
Class added when a node is being (touch) dragged over a dropZone.touchDropZoneClass?: string;
/**
* Function that is called when dragend event occurrs event occurs.
*/
MultiDragConfig<T>.handleDragend: NodeDragEventData<T> | NodeTouchEventData<T>
Function that is called when dragend event occurrs event occurs.handleDragend: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in MultiDragConfig<T>
T> | interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* Function that is called when dragstart occurs.
*/
MultiDragConfig<T>.handleDragstart: NodeDragEventData<T>
Function that is called when dragstart occurs.handleDragstart: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* Function that is called when dragstart event occurs.
*/
MultiDragConfig<T>.handleTouchstart: NodeTouchEventData<T>
Function that is called when dragstart event occurs.handleTouchstart: interface NodeTouchEventData<T>
The data passed to the node event listener when the event is a touch event.NodeTouchEventData<function (type parameter) T in MultiDragConfig<T>
T>;
/**
* An array of functions to use for a given parent.
*/
MultiDragConfig<T>.plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins?: interface Array<T>
Array<type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin>;
}
Support Us
FormKit Drag and Drop is made with love by the FormKit team — the creators of open-source projects such as:
- FormKit - The open-source form framework for Vue.
- AutoAnimate - Add motion to your apps with a single line of code.
- Tempo - The easiest way to work with dates in JavaScript.
- ArrowJS - Reactivity without the Framework.
If you find our projects useful and would like to support their ongoing development, please consider sponsoring us on GitHub!