AI-Generated forms in seconds.
KickStart your next FormKit form in seconds. Generate from a prompt, image, or text attachment. Copy & paste as Vue components or FormKit schema.
Introduction
FormKit’s Drag and Drop is a small library for adding drag and drop functionality to your app. It’s simple, flexible, framework agnostic, and clocks in at only ~5kb gzipped. Drag and Drop works with React, Solid, Vue, Svelte, or any JavaScript framework. This library differs from others in the way the drag and drop operations are performed. Rather than moving elements around the DOM manually, Drag and Drop updates the reactive data model used by the provided render function.
Getting Started
Install
Drag and Drop is published as @formkit/drag-and-drop
on npm. Click to copy the install command for your package manager of choice:
- npm install @formkit/drag-and-drop
- pnpm add @formkit/drag-and-drop
- yarn add @formkit/drag-and-drop
- bun install @formkit/drag-and-drop
Usage
Drag and drop ships two main functions: dragAndDrop
and useDragAndDrop
. These can be imported for your framework of choice by using the subpath import @formkit/drag-and-drop/{react/vue}
. A native JavaScript version of the dragAndDrop
function is also available at @formkit/drag-and-drop
.
Whichever method you choose, the core concept remains the same: FormKit's Drag and Drop accepts two main arguments — the parent element containing the draggable items and the data that those items represent.
useDragAndDrop
The useDragAndDrop
hook is the most convenient way to add Drag and Drop to your app and is available for React and Vue. Call this function with an initial array of values and the configuration options. It returns an array of values containing a a template ref, a reactive set of values (and a setter in the case of React), as well as a function to update the parent's config. The template ref should be assigned to the parent DOM element of the items you wish to make draggable. The reactive array of values should be used in your template to render the draggable elements.
// Example parent configuration object:const config = { sortable: false }
// React:const [parentRef, values, setValues, updateConfig] = useDragAndDrop(
['Item 1', 'Item2', 'Item3'],
config
)
// Vue:const [parentRef, values, updateConfig] = useDragAndDrop(
['Item 1', 'Item2', 'Item3'],
config
)
dragAndDrop
The dragAndDrop
hook allows you to define your own ref and list state. This is useful if you need to integrate with a pre-existing app without changing your component structure.
// Example parent configuration object:const config = { sortable: false }
// React:dragAndDrop({
parent: parentRef,
state: [values, setValues],
config
})
// Vue:dragAndDrop({
parent: parentRef,
values: valueRef,
config
})
// Vanilla JS: (import from core, not subpath)
dragAndDrop({
parent: HTMLElement,
getValues: () => {
// Return array of values
},
setValues: (newValues) =>
// Do something with updated values
},
config
})
Core Features
Sortability
Using useDragAndDrop
or dragAndDrop
automatically makes a lists sortable. Dragging items within your list will automatically change your list’s state to reflect the new order (which in turn allows the framework to re-render to the correct order):
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
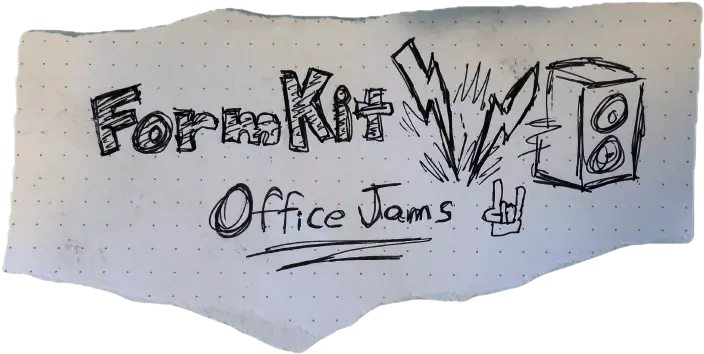
- Depeche Mode
- Duran Duran
- Pet Shop Boys
- Kraftwerk
- Tears for Fears
- Spandau Ballet
["Depeche Mode","Duran Duran","Pet Shop Boys","Kraftwerk","Tears for Fears","Spandau Ballet"]
Draggable
For drag-and-drop to function correctly, the length of array of values provided must be equivalent to the number of immediate children to the parent element. If they are not, a console warning will appear: The number of draggable items in the parent does not match the number of values, which may lead to unexpected behavior."
The most common cause of this is when the parent element has an immediate child that is not meant to be draggable. In this case, the configuration property draggable
can be set to a callback function that lets you determine whether or not a given element should be draggable:
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.id?: string | undefined
id="no-drag">I am NOT draggable</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.id?: string | undefined
id="no-drag">I am NOT draggable</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
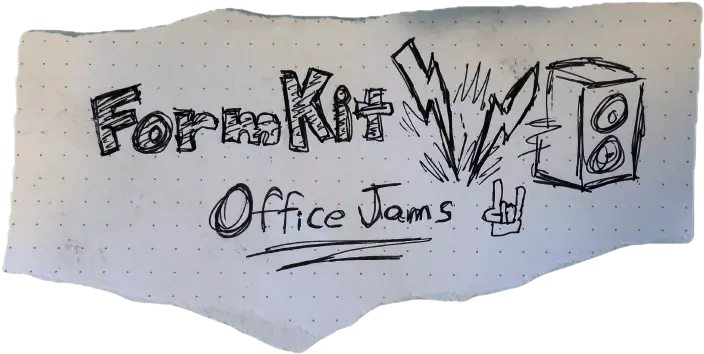
- Depeche Mode
- Duran Duran
- Pet Shop Boys
- Kraftwerk
- Tears for Fears
- Spandau Ballet
- I am NOT draggable
["Depeche Mode","Duran Duran","Pet Shop Boys","Kraftwerk","Tears for Fears","Spandau Ballet"]
Transferability
In addition to sorting, drag-and-drop allows transferring values between parents (lists). To enable this, set the configuration property group
to the same value for each list that should allow value transfers. Notice in the example below that items can be appended to the end of the list by hovering over the list itself, or inserted into the list by hovering over the target parent's draggable items.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = [
"Schedule perm",
"Rewind VHS tapes",
"Make change for the arcade",
"Get disposable camera developed",
"Learn C++",
"Return Nintendo Power Glove",
];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = [
"Schedule perm",
"Rewind VHS tapes",
"Make change for the arcade",
"Get disposable camera developed",
"Learn C++",
"Return Nintendo Power Glove",
];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{ group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList" }
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
["Schedule perm","Rewind VHS tapes","Make change for the arcade","Get disposable camera developed","Learn C++","Return Nintendo Power Glove"]
Complete
- Pickup new mix-tape from Beth
["Pickup new mix-tape from Beth"]
Disable sort
Sortability within a given parent can be toggled on and off by setting the sortable
property to in the configuration.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable: false
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
["Schedule perm","Rewind VHS tapes","Make change for the arcade","Get disposable camera developed","Learn C++","Return Nintendo Power Glove"]
Complete
- Pickup new mix-tape from Beth
["Pickup new mix-tape from Beth"]
Accepts
For a more nuanced transfer experience, define the accepts
property in the configuration. This property is a callback function that lets you determine whether or not a given element can be transferred into the given list.
- React
- Vue
- Native
import React from "react";
import type { interface ParentConfig<T>
The configuration object for a given parent.ParentConfig } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export default function function myComponent(): React.JSX.Element
myComponent() {
const [const source: React.RefObject<HTMLUListElement>
source, const items1: string[]
items1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["dungeon_master.exe", "map_1.dat", "map_2.dat", "character1.txt", "character2.txt", "shell32.dll", "README.txt",],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
const const config1: Partial<ParentConfig<string>>
config1: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config1: Partial<ParentConfig<string>>
config1.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, currentParentData: ParentRecord<string>, state: BaseDragState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target2: React.RefObject<HTMLElement>
target2.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items2: string[]
items2.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 3;
};
const const config2: Partial<ParentConfig<string>>
config2: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config2: Partial<ParentConfig<string>>
config2.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, currentParentData: ParentRecord<string>, state: BaseDragState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target1: React.RefObject<HTMLElement>
target1.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items3: string[]
items3.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 5;
};
const [const target1: React.RefObject<HTMLElement>
target1, const items2: string[]
items2] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["knight.bmp", "dragon.bmp"], const config1: Partial<ParentConfig<string>>
config1);
const [const target2: React.RefObject<HTMLElement>
target2, const items3: string[]
items3] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["brick.bmp", "moss.bmp"], const config2: Partial<ParentConfig<string>>
config2);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const source: React.RefObject<HTMLUListElement>
source}>
{const items1: string[]
items1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target1">
{const items2: string[]
items2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target2">
{const items3: string[]
items3.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import type { interface ParentConfig<T>
The configuration object for a given parent.ParentConfig } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export default function function myComponent(): React.JSX.Element
myComponent() {
const [const source: React.RefObject<HTMLUListElement>
source, const items1: string[]
items1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["dungeon_master.exe", "map_1.dat", "map_2.dat", "character1.txt", "character2.txt", "shell32.dll", "README.txt",],
{
draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable: (el: HTMLElement
el) => {
return el: HTMLElement
el.Element.id: string
Returns the value of element's id content attribute. Can be set to change it.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/id)id !== "no-drag";
},
}
);
const const config1: Partial<ParentConfig<string>>
config1: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config1: Partial<ParentConfig<string>>
config1.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, currentParentData: ParentRecord<string>, state: BaseDragState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target2: React.RefObject<HTMLElement>
target2.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items2: string[]
items2.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 3;
};
const const config2: Partial<ParentConfig<string>>
config2: type Partial<T> = { [P in keyof T]?: T[P] | undefined; }
Make all properties in T optionalPartial<interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<string>> = {};
const config2: Partial<ParentConfig<string>>
config2.accepts?: ((targetParentData: ParentRecord<string>, initialParentData: ParentRecord<string>, currentParentData: ParentRecord<string>, state: BaseDragState<...>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts = (_parent: ParentRecord<string>
_parent, lastParent: ParentRecord<string>
lastParent) => {
if (lastParent: ParentRecord<string>
lastParent.ParentRecord<string>.el: HTMLElement
el === const target1: React.RefObject<HTMLElement>
target1.React.RefObject<HTMLElement>.current: HTMLElement | null
The current value of the ref.current) {
return false;
}
return const items3: string[]
items3.Array<string>.length: number
Gets or sets the length of the array. This is a number one higher than the highest index in the array.length < 5;
};
const [const target1: React.RefObject<HTMLElement>
target1, const items2: string[]
items2] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["knight.bmp", "dragon.bmp"], const config1: Partial<ParentConfig<string>>
config1);
const [const target2: React.RefObject<HTMLElement>
target2, const items3: string[]
items3] = useDragAndDrop<HTMLElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop(["brick.bmp", "moss.bmp"], const config2: Partial<ParentConfig<string>>
config2);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const source: React.RefObject<HTMLUListElement>
source}>
{const items1: string[]
items1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target1">
{const items2: string[]
items2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref="target2">
{const items3: string[]
items3.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
- dungeon_master.exe
- map_1.dat
- map_2.dat
- character1.txt
- character2.txt
- shell32.dll
- README.txt
- knight.bmp
- dragon.bmp
- brick.bmp
- moss.bmp
Drag Handles
Drag handles are a common way to allow users to drag items without accidentally dragging them when they don't intend to. To implement drag handles, set the dragHandle
property to a selector that will be used to find the handle.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth", "Implement drag handles"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the
draggable element.dragHandle: ".kanban-handle"
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the
draggable element.dragHandle: ".kanban-handle"
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = ["Schedule perm", "Rewind VHS tapes", "Make change for the arcade", "Get disposable camera developed", "Learn C++", "Return Nintendo Power Glove"];
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth", "Implement drag handles"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the
draggable element.dragHandle: ".kanban-handle"
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the
draggable element.dragHandle: ".kanban-handle"
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList} React.HTMLAttributes<T>.className?: string | undefined
className="kanban-column">
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span React.HTMLAttributes<T>.className?: string | undefined
className="kanban-handle"></JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
["Schedule perm","Rewind VHS tapes","Make change for the arcade","Get disposable camera developed","Learn C++","Return Nintendo Power Glove"]
Complete
- Pickup new mix-tape from Beth
- Implement drag handles
["Pickup new mix-tape from Beth","Implement drag handles"]
Updating Config
The parent's configuration can be updated idempotently during runtime by using the updateConfig
method. In the example below, clicking the button will toggle whether drag and drop is enabled for the parent.
- React
- Vue
- Native
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, React.Dispatch<React.SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const values: string[]
values, const _setValues: React.Dispatch<React.SetStateAction<string[]>>
_setValues, const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<
HTMLUListElement,
string
>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
const [const disabled: boolean
disabled, const setDisabled: React.Dispatch<React.SetStateAction<boolean>>
setDisabled] = useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const toggleDisabled: () => void
toggleDisabled = () => {
const setDisabled: (value: React.SetStateAction<boolean>) => void
setDisabled(!const disabled: boolean
disabled);
const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig({ disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled: !const disabled: boolean
disabled });
};
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const values: string[]
values.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={const toggleDisabled: () => void
toggleDisabled}>
{const disabled: boolean
disabled ? "Enable" : "Disable"} drag and drop
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, React.Dispatch<React.SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const values: string[]
values, const _setValues: React.Dispatch<React.SetStateAction<string[]>>
_setValues, const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<
HTMLUListElement,
string
>([
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
]);
const [const disabled: boolean
disabled, const setDisabled: React.Dispatch<React.SetStateAction<boolean>>
setDisabled] = useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const toggleDisabled: () => void
toggleDisabled = () => {
const setDisabled: (value: React.SetStateAction<boolean>) => void
setDisabled(!const disabled: boolean
disabled);
const updateConfig: (config: Partial<ParentConfig<string>>) => void
updateConfig({ disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled: !const disabled: boolean
disabled });
};
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const values: string[]
values.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={const toggleDisabled: () => void
toggleDisabled}>
{const disabled: boolean
disabled ? "Enable" : "Disable"} drag and drop
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
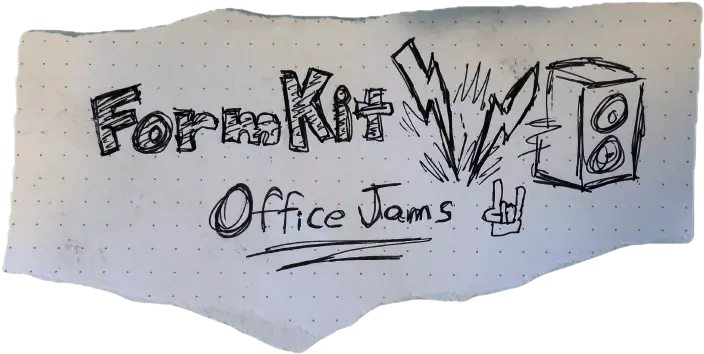
- Depeche Mode
- Duran Duran
- Pet
- Kraftwerk
- Tears for Fears
- Spandau Ballet
Multi Drag
Multi drag allows users to select multiple items and drag them all at once. To enable multi drag, set the multiDrag
property to true
in the configuration.
- React
- Vue
- Native
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const mockFileNames: string[]
mockFileNames = [
"dungeon_master.exe",
"map_1.dat",
"map_2.dat",
"character1.txt",
"character2.txt",
"shell32.dll",
"README.txt",
];
const [const parent1: React.RefObject<HTMLUListElement>
parent1, const files1: string[]
files1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const mockFileNames: string[]
mockFileNames,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
multiDrag?: boolean | undefined
multiDrag: true,
selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
}
);
const [const parent2: React.RefObject<HTMLUListElement>
parent2, const files2: string[]
files2] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([], {
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
multiDrag?: boolean | undefined
multiDrag: true,
selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
});
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="file-manager">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent1: React.RefObject<HTMLUListElement>
parent1} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files1: string[]
files1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">
{file: string
file}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent2: React.RefObject<HTMLUListElement>
parent2} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files2: string[]
files2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">
{file: string
file}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const mockFileNames: string[]
mockFileNames = [
"dungeon_master.exe",
"map_1.dat",
"map_2.dat",
"character1.txt",
"character2.txt",
"shell32.dll",
"README.txt",
];
const [const parent1: React.RefObject<HTMLUListElement>
parent1, const files1: string[]
files1] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const mockFileNames: string[]
mockFileNames,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
multiDrag?: boolean | undefined
multiDrag: true,
selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
}
);
const [const parent2: React.RefObject<HTMLUListElement>
parent2, const files2: string[]
files2] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([], {
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "A",
multiDrag?: boolean | undefined
multiDrag: true,
selectedClass?: string | undefined
selectedClass: "bg-blue-500 text-white",
});
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="file-manager">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent1: React.RefObject<HTMLUListElement>
parent1} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files1: string[]
files1.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">
{file: string
file}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent2: React.RefObject<HTMLUListElement>
parent2} React.HTMLAttributes<T>.className?: string | undefined
className="file-list">
{const files2: string[]
files2.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((file: string
file) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={file: string
file} React.HTMLAttributes<T>.className?: string | undefined
className="file">
{file: string
file}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
- dungeon_master.exe
- map_1.dat
- map_2.dat
- character1.txt
- character2.txt
- shell32.dll
- README.txt
Plugins
Plugins are a powerful way to extend the functionality of the library. They can be used to add new features, modify existing ones, or even create entirely new experiences.
Drop or Swap
The examples seen so far have shown the values of a given list being updated as the end-user drags over the list and its children. To enable behavior where the values of the list are only updated when the user drops the item, use the dropOrSwap
plugin.
- React
- Vue
- Native
import React from "react";
import { function dropOrSwap<T>(dropSwapConfig?: DropSwapConfig<T>): (parent: HTMLElement) => {
setup(): void;
} | undefined
dropOrSwap } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = [
"Schedule perm",
"Rewind VHS tapes",
"Make change for the arcade",
"Get disposable camera developed",
"Learn C++",
"Return Nintendo Power Glove",
];
const [const todoSwap: boolean
todoSwap, const setTodoSwap: React.Dispatch<React.SetStateAction<boolean>>
setTodoSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const [const doneSwap: boolean
doneSwap, const setDoneSwap: React.Dispatch<React.SetStateAction<boolean>>
setDoneSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
dropOrSwap<unknown>(dropSwapConfig?: DropSwapConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
} | undefined
dropOrSwap({
DropSwapConfig<unknown>.shouldSwap?: ((data: ShouldSwapData<unknown>) => boolean) | undefined
shouldSwap: () => {
return const todoSwap: boolean
todoSwap;
},
}),
],
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
dropOrSwap<unknown>(dropSwapConfig?: DropSwapConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
} | undefined
dropOrSwap({
DropSwapConfig<unknown>.shouldSwap?: ((data: ShouldSwapData<unknown>) => boolean) | undefined
shouldSwap: () => {
return const doneSwap: boolean
doneSwap;
},
}),
],
}
);
function function (local function) toggleTodoSwap(): void
toggleTodoSwap() {
const setTodoSwap: (value: React.SetStateAction<boolean>) => void
setTodoSwap(!const todoSwap: boolean
todoSwap);
}
function function (local function) toggleDoneSwap(): void
toggleDoneSwap() {
const setDoneSwap: (value: React.SetStateAction<boolean>) => void
setDoneSwap(!const doneSwap: boolean
doneSwap);
}
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={function (local function) toggleTodoSwap(): void
toggleTodoSwap}>
{" "}
Toggle {const todoSwap: boolean
todoSwap ? "Drop" : "Swap"}
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={function (local function) toggleDoneSwap(): void
toggleDoneSwap}>
Toggle {const doneSwap: boolean
doneSwap ? "Drop" : "Swap"}
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function dropOrSwap<T>(dropSwapConfig?: DropSwapConfig<T>): (parent: HTMLElement) => {
setup(): void;
} | undefined
dropOrSwap } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = [
"Schedule perm",
"Rewind VHS tapes",
"Make change for the arcade",
"Get disposable camera developed",
"Learn C++",
"Return Nintendo Power Glove",
];
const [const todoSwap: boolean
todoSwap, const setTodoSwap: React.Dispatch<React.SetStateAction<boolean>>
setTodoSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const [const doneSwap: boolean
doneSwap, const setDoneSwap: React.Dispatch<React.SetStateAction<boolean>>
setDoneSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
dropOrSwap<unknown>(dropSwapConfig?: DropSwapConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
} | undefined
dropOrSwap({
DropSwapConfig<unknown>.shouldSwap?: ((data: ShouldSwapData<unknown>) => boolean) | undefined
shouldSwap: () => {
return const todoSwap: boolean
todoSwap;
},
}),
],
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
dropOrSwap<unknown>(dropSwapConfig?: DropSwapConfig<unknown> | undefined): (parent: HTMLElement) => {
setup(): void;
} | undefined
dropOrSwap({
DropSwapConfig<unknown>.shouldSwap?: ((data: ShouldSwapData<unknown>) => boolean) | undefined
shouldSwap: () => {
return const doneSwap: boolean
doneSwap;
},
}),
],
}
);
function function (local function) toggleTodoSwap(): void
toggleTodoSwap() {
const setTodoSwap: (value: React.SetStateAction<boolean>) => void
setTodoSwap(!const todoSwap: boolean
todoSwap);
}
function function (local function) toggleDoneSwap(): void
toggleDoneSwap() {
const setDoneSwap: (value: React.SetStateAction<boolean>) => void
setDoneSwap(!const doneSwap: boolean
doneSwap);
}
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={function (local function) toggleTodoSwap(): void
toggleTodoSwap}>
{" "}
Toggle {const todoSwap: boolean
todoSwap ? "Drop" : "Swap"}
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button React.DOMAttributes<HTMLButtonElement>.onClick?: React.MouseEventHandler<HTMLButtonElement> | undefined
onClick={function (local function) toggleDoneSwap(): void
toggleDoneSwap}>
Toggle {const doneSwap: boolean
doneSwap ? "Drop" : "Swap"}
</JSX.IntrinsicElements.button: React.DetailedHTMLProps<React.ButtonHTMLAttributes<HTMLButtonElement>, HTMLButtonElement>
button>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
["Schedule perm","Rewind VHS tapes","Make change for the arcade","Get disposable camera developed","Learn C++","Return Nintendo Power Glove"]
Complete
- Pickup new mix-tape from Beth
["Pickup new mix-tape from Beth"]
Insert (Experimental)
Similar to the dropOrSwap
plugin, the insert
plugin does not update values until the user drops the item. In addition, the insert
plugin will render an insert point between the items to indicate where the item will be placed.
- React
- Vue
- Native
import React from "react";
import { function insert<T>(insertConfig: InsertConfig<T>): (parent: HTMLElement) => {
teardown(): void;
setup(): void;
} | undefined
insert } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
const const insertPointClasses: string[]
insertPointClasses = [
"absolute",
"bg-blue-500",
"z-[1000]",
"rounded-full",
"duration-[5ms]",
"before:block",
'before:content-["Insert"]',
"before:whitespace-nowrap",
"before:block",
"before:bg-blue-500",
"before:py-1",
"before:px-2",
"before:rounded-full",
"before:text-xs",
"before:absolute",
"before:top-1/2",
"before:left-1/2",
"before:-translate-y-1/2",
"before:-translate-x-1/2",
"before:text-white",
"before:text-xs",
];
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = [
"Schedule perm",
"Rewind VHS tapes",
"Make change for the arcade",
"Get disposable camera developed",
"Learn C++",
"Return Nintendo Power Glove",
];
const [const todoSwap: boolean
todoSwap, const setTodoSwap: React.Dispatch<React.SetStateAction<boolean>>
setTodoSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const [const doneSwap: boolean
doneSwap, const setDoneSwap: React.Dispatch<React.SetStateAction<boolean>>
setDoneSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
insert<unknown>(insertConfig: InsertConfig<unknown>): (parent: HTMLElement) => {
teardown(): void;
setup(): void;
} | undefined
insert({
InsertConfig<unknown>.insertPoint: (parent: ParentRecord<unknown>) => HTMLElement
insertPoint: (parent: ParentRecord<unknown>
parent) => {
const const div: HTMLDivElement
div = var document: Document
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Window/document)document.Document.createElement<"div">(tagName: "div", options?: ElementCreationOptions): HTMLDivElement (+2 overloads)
Creates an instance of the element for the specified tag.createElement("div");
for (const const cls: string
cls of const insertPointClasses: string[]
insertPointClasses) const div: HTMLDivElement
div.Element.classList: DOMTokenList
Allows for manipulation of element's class content attribute as a set of whitespace-separated tokens through a DOMTokenList object.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/classList)classList.DOMTokenList.add(...tokens: string[]): void
Adds all arguments passed, except those already present.
Throws a "SyntaxError" DOMException if one of the arguments is the empty string.
Throws an "InvalidCharacterError" DOMException if one of the arguments contains any ASCII whitespace.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/DOMTokenList/add)add(const cls: string
cls);
return const div: HTMLDivElement
div;
},
}),
],
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
insert<unknown>(insertConfig: InsertConfig<unknown>): (parent: HTMLElement) => {
teardown(): void;
setup(): void;
} | undefined
insert({
InsertConfig<unknown>.insertPoint: (parent: ParentRecord<unknown>) => HTMLElement
insertPoint: (parent: ParentRecord<unknown>
parent) => {
const const div: HTMLDivElement
div = var document: Document
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Window/document)document.Document.createElement<"div">(tagName: "div", options?: ElementCreationOptions): HTMLDivElement (+2 overloads)
Creates an instance of the element for the specified tag.createElement("div");
for (const const cls: string
cls of const insertPointClasses: string[]
insertPointClasses) const div: HTMLDivElement
div.Element.classList: DOMTokenList
Allows for manipulation of element's class content attribute as a set of whitespace-separated tokens through a DOMTokenList object.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/classList)classList.DOMTokenList.add(...tokens: string[]): void
Adds all arguments passed, except those already present.
Throws a "SyntaxError" DOMException if one of the arguments is the empty string.
Throws an "InvalidCharacterError" DOMException if one of the arguments contains any ASCII whitespace.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/DOMTokenList/add)add(const cls: string
cls);
return const div: HTMLDivElement
div;
},
}),
],
}
);
function function (local function) toggleTodoSwap(): void
toggleTodoSwap() {
const setTodoSwap: (value: React.SetStateAction<boolean>) => void
setTodoSwap(!const todoSwap: boolean
todoSwap);
}
function function (local function) toggleDoneSwap(): void
toggleDoneSwap() {
const setDoneSwap: (value: React.SetStateAction<boolean>) => void
setDoneSwap(!const doneSwap: boolean
doneSwap);
}
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function insert<T>(insertConfig: InsertConfig<T>): (parent: HTMLElement) => {
teardown(): void;
setup(): void;
} | undefined
insert } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
const const insertPointClasses: string[]
insertPointClasses = [
"absolute",
"bg-blue-500",
"z-[1000]",
"rounded-full",
"duration-[5ms]",
"before:block",
'before:content-["Insert"]',
"before:whitespace-nowrap",
"before:block",
"before:bg-blue-500",
"before:py-1",
"before:px-2",
"before:rounded-full",
"before:text-xs",
"before:absolute",
"before:top-1/2",
"before:left-1/2",
"before:-translate-y-1/2",
"before:-translate-x-1/2",
"before:text-white",
"before:text-xs",
];
export function function myComponent(): React.JSX.Element
myComponent() {
const const todoItems: string[]
todoItems = [
"Schedule perm",
"Rewind VHS tapes",
"Make change for the arcade",
"Get disposable camera developed",
"Learn C++",
"Return Nintendo Power Glove",
];
const [const todoSwap: boolean
todoSwap, const setTodoSwap: React.Dispatch<React.SetStateAction<boolean>>
setTodoSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const [const doneSwap: boolean
doneSwap, const setDoneSwap: React.Dispatch<React.SetStateAction<boolean>>
setDoneSwap] = React.function React.useState<boolean>(initialState: boolean | (() => boolean)): [boolean, React.Dispatch<React.SetStateAction<boolean>>] (+1 overload)
Returns a stateful value, and a function to update it.useState(false);
const const doneItems: string[]
doneItems = ["Pickup new mix-tape from Beth"];
const [const todoList: React.RefObject<HTMLUListElement>
todoList, const todos: string[]
todos] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const todoItems: string[]
todoItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
insert<unknown>(insertConfig: InsertConfig<unknown>): (parent: HTMLElement) => {
teardown(): void;
setup(): void;
} | undefined
insert({
InsertConfig<unknown>.insertPoint: (parent: ParentRecord<unknown>) => HTMLElement
insertPoint: (parent: ParentRecord<unknown>
parent) => {
const const div: HTMLDivElement
div = var document: Document
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Window/document)document.Document.createElement<"div">(tagName: "div", options?: ElementCreationOptions): HTMLDivElement (+2 overloads)
Creates an instance of the element for the specified tag.createElement("div");
for (const const cls: string
cls of const insertPointClasses: string[]
insertPointClasses) const div: HTMLDivElement
div.Element.classList: DOMTokenList
Allows for manipulation of element's class content attribute as a set of whitespace-separated tokens through a DOMTokenList object.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/classList)classList.DOMTokenList.add(...tokens: string[]): void
Adds all arguments passed, except those already present.
Throws a "SyntaxError" DOMException if one of the arguments is the empty string.
Throws an "InvalidCharacterError" DOMException if one of the arguments contains any ASCII whitespace.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/DOMTokenList/add)add(const cls: string
cls);
return const div: HTMLDivElement
div;
},
}),
],
}
);
const [const doneList: React.RefObject<HTMLUListElement>
doneList, const dones: string[]
dones] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
const doneItems: string[]
doneItems,
{
group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group: "todoList",
plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [
insert<unknown>(insertConfig: InsertConfig<unknown>): (parent: HTMLElement) => {
teardown(): void;
setup(): void;
} | undefined
insert({
InsertConfig<unknown>.insertPoint: (parent: ParentRecord<unknown>) => HTMLElement
insertPoint: (parent: ParentRecord<unknown>
parent) => {
const const div: HTMLDivElement
div = var document: Document
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Window/document)document.Document.createElement<"div">(tagName: "div", options?: ElementCreationOptions): HTMLDivElement (+2 overloads)
Creates an instance of the element for the specified tag.createElement("div");
for (const const cls: string
cls of const insertPointClasses: string[]
insertPointClasses) const div: HTMLDivElement
div.Element.classList: DOMTokenList
Allows for manipulation of element's class content attribute as a set of whitespace-separated tokens through a DOMTokenList object.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/Element/classList)classList.DOMTokenList.add(...tokens: string[]): void
Adds all arguments passed, except those already present.
Throws a "SyntaxError" DOMException if one of the arguments is the empty string.
Throws an "InvalidCharacterError" DOMException if one of the arguments contains any ASCII whitespace.
[MDN Reference](https://developer.mozilla.org/docs/Web/API/DOMTokenList/add)add(const cls: string
cls);
return const div: HTMLDivElement
div;
},
}),
],
}
);
function function (local function) toggleTodoSwap(): void
toggleTodoSwap() {
const setTodoSwap: (value: React.SetStateAction<boolean>) => void
setTodoSwap(!const todoSwap: boolean
todoSwap);
}
function function (local function) toggleDoneSwap(): void
toggleDoneSwap() {
const setDoneSwap: (value: React.SetStateAction<boolean>) => void
setDoneSwap(!const doneSwap: boolean
doneSwap);
}
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div React.HTMLAttributes<HTMLDivElement>.className?: string | undefined
className="kanban-board">
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const todoList: React.RefObject<HTMLUListElement>
todoList}>
{const todos: string[]
todos.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((todo: string
todo) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={todo: string
todo}>
{todo: string
todo}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const doneList: React.RefObject<HTMLUListElement>
doneList}>
{const dones: string[]
dones.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((done: string
done) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<T>.className?: string | undefined
className="kanban-item" React.Attributes.key?: React.Key | null | undefined
key={done: string
done}>
{done: string
done}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
ToDos
- Schedule perm
- Rewind VHS tapes
- Make change for the arcade
- Get disposable camera developed
- Learn C++
- Return Nintendo Power Glove
["Schedule perm","Rewind VHS tapes","Make change for the arcade","Get disposable camera developed","Learn C++","Return Nintendo Power Glove"]
Complete
- Pickup new mix-tape from Beth
["Pickup new mix-tape from Beth"]
Animations (Experimenal)
To add animations to your drag and drop experience, you can use the animations
plugin.
- React
- Vue
- Native
import React from "react";
import { function animations(animationsConfig?: Partial<AnimationsConfig>): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{ plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [function animations(animationsConfig?: Partial<AnimationsConfig>): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations()] }
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
import React from "react";
import { function animations(animationsConfig?: Partial<AnimationsConfig>): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function myComponent(): React.JSX.Element
myComponent() {
const [const parent: React.RefObject<HTMLUListElement>
parent, const tapes: string[]
tapes] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
[
"Depeche Mode",
"Duran Duran",
"Pet Shop Boys",
"Kraftwerk",
"Tears for Fears",
"Spandau Ballet",
],
{ plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins: [function animations(animationsConfig?: Partial<AnimationsConfig>): (parent: HTMLElement) => {
setup(): void;
setupNodeRemap<T>(data: SetupNodeData<T>): void;
} | undefined
animations()] }
);
return (
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const tapes: string[]
tapes.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((tape: string
tape) => (
<JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.HTMLAttributes<HTMLLIElement>.className?: string | undefined
className="cassette" data-label: string
data-label={tape: string
tape} React.Attributes.key?: React.Key | null | undefined
key={tape: string
tape}>
{tape: string
tape}
</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>
))}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
);
}
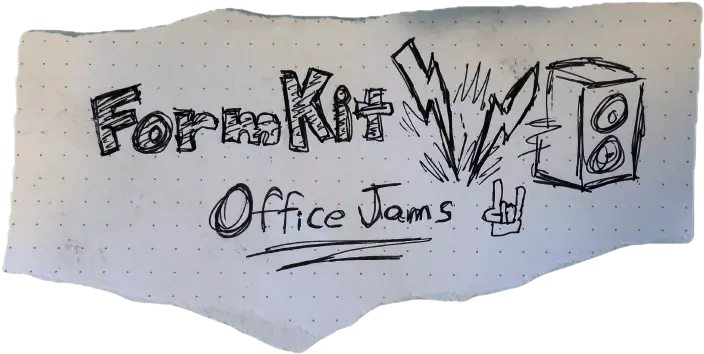
- Depeche Mode
- Duran Duran
- Pet Shop Boys
- Kraftwerk
- Tears for Fears
- Spandau Ballet
Events
When using a drag and drop library, often it is useful to be able to listen to particular events, such as when a drag starts or ends. Event listeners can be set at either a global level or at the parent level.
Global
Two global events are available, `dragStarted` and `dragEnded`. In order to listen to global events, import the state
object from @formkit/drag-and-drop
and register your event listener using the on
method.
- React
- Vue
- Native
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, React.Dispatch<React.SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { let state: BaseDragState<unknown>
The state of the drag and drop.state } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function App(): React.JSX.Element
App() {
const [const dragStatus: string
dragStatus, const setDragStatus: React.Dispatch<React.SetStateAction<string>>
setDragStatus] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not dragging");
const [const parent: React.RefObject<HTMLUListElement>
parent, const items: string[]
items] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([
"🍦 vanilla",
"🍫 chocolate",
"🍓 strawberry",
]);
let state: BaseDragState<unknown>
The state of the drag and drop.state.on: (event: string, callback: (data: unknown) => void) => void
on("dragStarted", () => const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Dragging"));
let state: BaseDragState<unknown>
The state of the drag and drop.state.on: (event: string, callback: (data: unknown) => void) => void
on("dragEnded", () => const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Not dragging"));
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>Rank your favorite flavors</JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragStatus: string
dragStatus}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const items: string[]
items.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => {
return <JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>;
})}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, React.Dispatch<React.SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { let state: BaseDragState<unknown>
The state of the drag and drop.state } from "@formkit/drag-and-drop";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function App(): React.JSX.Element
App() {
const [const dragStatus: string
dragStatus, const setDragStatus: React.Dispatch<React.SetStateAction<string>>
setDragStatus] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not dragging");
const [const parent: React.RefObject<HTMLUListElement>
parent, const items: string[]
items] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>([
"🍦 vanilla",
"🍫 chocolate",
"🍓 strawberry",
]);
let state: BaseDragState<unknown>
The state of the drag and drop.state.on: (event: string, callback: (data: unknown) => void) => void
on("dragStarted", () => const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Dragging"));
let state: BaseDragState<unknown>
The state of the drag and drop.state.on: (event: string, callback: (data: unknown) => void) => void
on("dragEnded", () => const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Not dragging"));
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>Rank your favorite flavors</JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragStatus: string
dragStatus}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const items: string[]
items.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => {
return <JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>;
})}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
Parent
Parent's themselves have their own set of events that can be listened to. These events include: `onDragstart`, `onDragend`, `onSort`, `onTransfer`. To listen to parent events, assign a callback function to the event in the parent's configuration object.
- React
- Vue
- Native
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, React.Dispatch<React.SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function App(): React.JSX.Element
App() {
const [const dragStatus: string
dragStatus, const setDragStatus: React.Dispatch<React.SetStateAction<string>>
setDragStatus] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not dragging");
const [const valuesChanged: string
valuesChanged, const setValuesChanged: React.Dispatch<React.SetStateAction<string>>
setValuesChanged] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not sorting");
const [const parent: React.RefObject<HTMLUListElement>
parent, const items: string[]
items] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["🍦 vanilla", "🍫 chocolate", "🍓 strawberry"],
{
onDragstart?: DragstartEvent | undefined
Fired when a drag is started, whether native drag or syntheticonDragstart: () => {
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Dragging");
},
onDragend?: DragendEvent | undefined
Fired when a drag is ended, whether native drag or syntheticonDragend: () => {
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Not dragging");
const setValuesChanged: (value: React.SetStateAction<string>) => void
setValuesChanged("Not sorting");
},
onSort?: SortEvent | undefined
Callback function for when a sort operation is performed.onSort: (event: SortEventData<T>
event) => {
const setValuesChanged: (value: React.SetStateAction<string>) => void
setValuesChanged(`${event: SortEventData<T>
event.SortEventData<T>.previousValues: T[]
previousValues} -> ${event: SortEventData<T>
event.SortEventData<T>.values: T[]
values}`);
},
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>Rank your favorite flavors</JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragStatus: string
dragStatus}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const valuesChanged: string
valuesChanged}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const items: string[]
items.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => {
return <JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>;
})}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
import React from "react";
import { function useState<S>(initialState: S | (() => S)): [S, React.Dispatch<React.SetStateAction<S>>] (+1 overload)
Returns a stateful value, and a function to update it.useState } from "react";
import { function useDragAndDrop<E extends HTMLElement, T = unknown>(list: T[], options?: Partial<ParentConfig<T>>): [React.RefObject<E>, T[], React.Dispatch<React.SetStateAction<T[]>>, (config: Partial<ParentConfig<...>>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop } from "@formkit/drag-and-drop/react";
export function function App(): React.JSX.Element
App() {
const [const dragStatus: string
dragStatus, const setDragStatus: React.Dispatch<React.SetStateAction<string>>
setDragStatus] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not dragging");
const [const valuesChanged: string
valuesChanged, const setValuesChanged: React.Dispatch<React.SetStateAction<string>>
setValuesChanged] = useState<string>(initialState: string | (() => string)): [string, React.Dispatch<React.SetStateAction<string>>] (+1 overload)
Returns a stateful value, and a function to update it.useState("Not sorting");
const [const parent: React.RefObject<HTMLUListElement>
parent, const items: string[]
items] = useDragAndDrop<HTMLUListElement, string>(list: string[], options?: Partial<ParentConfig<string>> | undefined): [React.RefObject<HTMLUListElement>, string[], React.Dispatch<...>, (config: Partial<...>) => void]
Hook for adding drag and drop/sortable support to a list of items.useDragAndDrop<HTMLUListElement, string>(
["🍦 vanilla", "🍫 chocolate", "🍓 strawberry"],
{
onDragstart?: DragstartEvent | undefined
Fired when a drag is started, whether native drag or syntheticonDragstart: () => {
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Dragging");
},
onDragend?: DragendEvent | undefined
Fired when a drag is ended, whether native drag or syntheticonDragend: () => {
const setDragStatus: (value: React.SetStateAction<string>) => void
setDragStatus("Not dragging");
const setValuesChanged: (value: React.SetStateAction<string>) => void
setValuesChanged("Not sorting");
},
onSort?: SortEvent | undefined
Callback function for when a sort operation is performed.onSort: (event: SortEventData<T>
event) => {
const setValuesChanged: (value: React.SetStateAction<string>) => void
setValuesChanged(`${event: SortEventData<T>
event.SortEventData<T>.previousValues: T[]
previousValues} -> ${event: SortEventData<T>
event.SortEventData<T>.values: T[]
values}`);
},
}
);
return (
<JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
<JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>Rank your favorite flavors</JSX.IntrinsicElements.strong: React.DetailedHTMLProps<React.HTMLAttributes<HTMLElement>, HTMLElement>
strong>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const dragStatus: string
dragStatus}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>{const valuesChanged: string
valuesChanged}</JSX.IntrinsicElements.span: React.DetailedHTMLProps<React.HTMLAttributes<HTMLSpanElement>, HTMLSpanElement>
span>
<JSX.IntrinsicElements.br: React.DetailedHTMLProps<React.HTMLAttributes<HTMLBRElement>, HTMLBRElement>
br />
<JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul React.RefAttributes<HTMLUListElement>.ref?: React.LegacyRef<HTMLUListElement> | undefined
Allows getting a ref to the component instance.
Once the component unmounts, React will set `ref.current` to `null`
(or call the ref with `null` if you passed a callback ref).ref={const parent: React.RefObject<HTMLUListElement>
parent}>
{const items: string[]
items.Array<string>.map<React.JSX.Element>(callbackfn: (value: string, index: number, array: string[]) => React.JSX.Element, thisArg?: any): React.JSX.Element[]
Calls a defined callback function on each element of an array, and returns an array that contains the results.map((item: string
item) => {
return <JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li React.Attributes.key?: React.Key | null | undefined
key={item: string
item}>{item: string
item}</JSX.IntrinsicElements.li: React.DetailedHTMLProps<React.LiHTMLAttributes<HTMLLIElement>, HTMLLIElement>
li>;
})}
</JSX.IntrinsicElements.ul: React.DetailedHTMLProps<React.HTMLAttributes<HTMLUListElement>, HTMLUListElement>
ul>
</JSX.IntrinsicElements.div: React.DetailedHTMLProps<React.HTMLAttributes<HTMLDivElement>, HTMLDivElement>
div>
);
}
Configuration
Each parent can be passed a configuration object. This object can be used to set options like group
, drag handles
, and or more dynamic options such as determining which direct descendants of a list are draggable
. Invocation of useDragAndDrop
or dragAndDrop
can be used idempotently to update the configuration for a given list.
import type {
interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord,
type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState,
interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData,
interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData,
interface NodeEventData<T>
The data passed to the node event listener.NodeEventData,
type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin,
interface PointeroverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.PointeroverNodeEvent,
type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode,
interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData,
type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode,
interface PointeroverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.PointeroverParentEvent,
type SortEvent = <T>(data: SortEventData<T>) => void
SortEvent,
type TransferEvent = <T>(data: TransferEventData<T>) => void
TransferEvent,
type DragstartEvent = <T>(data: DragstartEventData<T>) => void
DragstartEvent,
type DragendEvent = <T>(data: DragendEventData<T>) => void
DragendEvent,
interface ParentDragEventData<T>
ParentDragEventData,
type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState,
type NativeDragEffects = "link" | "none" | "copy" | "move"
NativeDragEffects,
interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord,
interface DropSwapConfig<T>
DropSwapConfig,
interface InsertConfig<T>
InsertConfig,
interface ParentData<T>
The data assigned to a given parent in the `parents` weakmap.ParentData,
interface ParentKeydownEventData<T>
ParentKeydownEventData,
type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState,
} from "@formkit/drag-and-drop";
/**
* The configuration object for a given parent.
*/
export interface interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<function (type parameter) T in ParentConfig<T>
T> {
/**
* A function that returns whether a given parent accepts a given node.
*/
ParentConfig<T>.accepts?: ((targetParentData: ParentRecord<T>, initialParentData: ParentRecord<T>, currentParentData: ParentRecord<T>, state: BaseDragState<T>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts?: (
targetParentData: ParentRecord<T>
targetParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
initialParentData: ParentRecord<T>
initialParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
currentParentData: ParentRecord<T>
currentParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T>
state: type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T>
) => boolean;
ParentConfig<T>.activeDescendantClass?: string | undefined
activeDescendantClass?: string;
/**
* The data transfer effect to use for the drag operation.
*/
ParentConfig<T>.dragEffectAllowed: NativeDragEffects
The data transfer effect to use for the drag operation.dragEffectAllowed: type NativeDragEffects = "link" | "none" | "copy" | "move"
NativeDragEffects;
/**
* The data transfer effect to use for the drag operation.
*/
ParentConfig<T>.dragDropEffect: NativeDragEffects
The data transfer effect to use for the drag operation.dragDropEffect: type NativeDragEffects = "link" | "none" | "copy" | "move"
NativeDragEffects;
/**
* A function that returns the image to use for the drag operation. This is
* invoked for native operations. The clonedNode is what will be set as the drag
* image, but this can be updated.
*/
ParentConfig<T>.dragImage?: ((data: NodeDragEventData<T>, draggedNodes: Array<NodeRecord<T>>) => HTMLElement) | undefined
A function that returns the image to use for the drag operation. This is
invoked for native operations. The clonedNode is what will be set as the drag
image, but this can be updated.dragImage?: (
data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>,
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>
) => HTMLElement;
/**
* A flag to disable dragability of all nodes in the parent.
*/
ParentConfig<T>.disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled?: boolean;
/**
* A selector for the drag handle. Will search at any depth within the
* draggable element.
*/
ParentConfig<T>.dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the
draggable element.dragHandle?: string;
/**
* External drag handle
*/
ParentConfig<T>.externalDragHandle?: {
el: HTMLElement;
callback: () => HTMLElement;
} | undefined
External drag handleexternalDragHandle?: {
el: HTMLElement
el: HTMLElement;
callback: () => HTMLElement
callback: () => HTMLElement;
};
/**
* A function that returns whether a given node is draggable.
*/
ParentConfig<T>.draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable?: (child: HTMLElement
child: HTMLElement) => boolean;
/**
* A function that returns whether a given value is draggable
*/
ParentConfig<T>.draggableValue?: ((values: T) => boolean) | undefined
A function that returns whether a given value is draggabledraggableValue?: (values: T
values: function (type parameter) T in ParentConfig<T>
T) => boolean;
ParentConfig<T>.draggedNodes: (pointerDown: {
parent: ParentRecord<T>;
node: NodeRecord<T>;
}) => Array<NodeRecord<T>>
draggedNodes: (pointerDown: {
parent: ParentRecord<T>;
node: NodeRecord<T>;
}
pointerDown: {
parent: ParentRecord<T>
parent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
node: NodeRecord<T>
node: interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>;
}) => interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
/**
* The class to add to a node when it is being dragged.
*/
ParentConfig<T>.draggingClass?: string | undefined
The class to add to a node when it is being dragged.draggingClass?: string;
/**
* Accepts array of "dragged nodes" and applies dragstart classes to them.
*/
ParentConfig<T>.dragstartClasses: (node: NodeRecord<T>, nodes: Array<NodeRecord<T>>, config: ParentConfig<T>, isSynthDrag?: boolean) => void
Accepts array of "dragged nodes" and applies dragstart classes to them.dragstartClasses: (
node: NodeRecord<T>
node: interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>,
nodes: NodeRecord<T>[]
nodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>,
config: ParentConfig<T>
config: interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<function (type parameter) T in ParentConfig<T>
T>,
isSynthDrag: boolean | undefined
isSynthDrag?: boolean
) => void;
// When drag starts, this will be applied to the dragged node(s) (not their
// representations being dragged) on dragstart. This will remain on the nodes
// until the drag ends.
ParentConfig<T>.dragPlaceholderClass?: string | undefined
dragPlaceholderClass?: string;
/**
* The configuration object for the drop and swap plugin.
*/
ParentConfig<T>.dropSwapConfig?: DropSwapConfig<T> | undefined
The configuration object for the drop and swap plugin.dropSwapConfig?: interface DropSwapConfig<T>
DropSwapConfig<function (type parameter) T in ParentConfig<T>
T>;
/**
* The class to add to a node when the node is dragged over it.
*/
ParentConfig<T>.dropZoneClass?: string | undefined
The class to add to a node when the node is dragged over it.dropZoneClass?: string;
/**
* The class to add to a parent when it is dragged over.
*/
ParentConfig<T>.dropZoneParentClass?: string | undefined
The class to add to a parent when it is dragged over.dropZoneParentClass?: string;
/**
* A flag to indicate whether the parent itself is a dropZone.
*/
ParentConfig<T>.dropZone?: boolean | undefined
A flag to indicate whether the parent itself is a dropZone.dropZone?: boolean;
/**
* The group that the parent belongs to. This is used for allowing multiple
* parents to transfer nodes between each other.
*/
ParentConfig<T>.group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group?: string;
ParentConfig<T>.handleParentFocus: (data: ParentEventData<T>, state: BaseDragState<T>) => void
handleParentFocus: (
data: ParentEventData<T>
data: interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T>
state: type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
ParentConfig<T>.handleNodeKeydown: (data: NodeEventData<T>, state: DragState<T>) => void
handleNodeKeydown: (data: NodeEventData<T>
data: interface NodeEventData<T>
The data passed to the node event listener.NodeEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleParentKeydown: (data: ParentKeydownEventData<T>, state: DragState<T>) => void
handleParentKeydown: (
data: ParentKeydownEventData<T>
data: interface ParentKeydownEventData<T>
ParentKeydownEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when dragend or touchend event occurs.
*/
ParentConfig<T>.handleDragend: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when dragend or touchend event occurs.handleDragend: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when dragstart event occurs.
*/
ParentConfig<T>.handleDragstart: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when dragstart event occurs.handleDragstart: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleEnd: (state: DragState<T> | SynthDragState<T>) => void
handleEnd: (state: DragState<T> | SynthDragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleNodeDrop: (data: NodeDragEventData<T>, state: DragState<T>) => void
handleNodeDrop: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleNodePointerup: (data: NodePointerEventData<T>, state: DragState<T>) => void
handleNodePointerup: (
data: NodePointerEventData<T>
data: interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
ParentConfig<T>.handleParentScroll: (data: ParentEventData<T>, state: DragState<T> | BaseDragState<T> | SynthDragState<T>) => void
handleParentScroll: (
data: ParentEventData<T>
data: interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a dragenter event is triggered on the node.
*/
ParentConfig<T>.handleNodeDragenter: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when a dragenter event is triggered on the node.handleNodeDragenter: (
data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Dragleave event on node
*/
ParentConfig<T>.handleNodeDragleave: (data: NodeDragEventData<T>, state: DragState<T>) => void
Dragleave event on nodehandleNodeDragleave: (
data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a dragover event is triggered on the parent.
*/
ParentConfig<T>.handleParentDragover: (data: ParentDragEventData<T>, state: DragState<T>) => void
Function that is called when a dragover event is triggered on the parent.handleParentDragover: (
data: ParentDragEventData<T>
data: interface ParentDragEventData<T>
ParentDragEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Drop event on parent
*/
ParentConfig<T>.handleParentDrop: (data: ParentDragEventData<T>, state: DragState<T>) => void
Drop event on parenthandleParentDrop: (data: ParentDragEventData<T>
data: interface ParentDragEventData<T>
ParentDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a dragover event is triggered on a node.
*/
ParentConfig<T>.handleNodeDragover: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when a dragover event is triggered on a node.handleNodeDragover: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handlePointercancel: (data: NodeDragEventData<T> | NodePointerEventData<T>, state: DragState<T> | SynthDragState<T> | BaseDragState<T>) => void
handlePointercancel: (
data: NodeDragEventData<T> | NodePointerEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T> | interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T> | type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/*
* Function that is called when a pointerdown is triggered on node.
*/
ParentConfig<T>.handleNodePointerdown: (data: NodePointerEventData<T>, state: DragState<T>) => void
handleNodePointerdown: (
data: NodePointerEventData<T>
data: interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over a given node (similar to dragover).
*/
ParentConfig<T>.handleNodePointerover: (data: PointeroverNodeEvent<T>, state: SynthDragState<T>) => void
Function that is called when a node that is being moved by touchmove event
is over a given node (similar to dragover).handleNodePointerover: (
data: PointeroverNodeEvent<T>
data: interface PointeroverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.PointeroverNodeEvent<function (type parameter) T in ParentConfig<T>
T>,
state: SynthDragState<T>
state: type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over the parent (similar to dragover).
*/
ParentConfig<T>.handleParentPointerover: (e: PointeroverParentEvent<T>, state: SynthDragState<T>) => void
Function that is called when a node that is being moved by touchmove event
is over the parent (similar to dragover).handleParentPointerover: (
e: PointeroverParentEvent<T>
e: interface PointeroverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.PointeroverParentEvent<function (type parameter) T in ParentConfig<T>
T>,
state: SynthDragState<T>
state: type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Config option for insert plugin.
*/
ParentConfig<T>.insertConfig?: InsertConfig<T> | undefined
Config option for insert plugin.insertConfig?: interface InsertConfig<T>
InsertConfig<function (type parameter) T in ParentConfig<T>
T>;
/**
* A flag to indicate whether long touch is enabled.
*/
ParentConfig<T>.longPress?: boolean | undefined
A flag to indicate whether long touch is enabled.longPress?: boolean;
/**
* The class to add to a node when a long touch action is performed.
*/
ParentConfig<T>.longPressClass?: string | undefined
The class to add to a node when a long touch action is performed.longPressClass?: string;
/**
* The time in milliseconds to wait before a long touch is performed.
*/
ParentConfig<T>.longPressDuration?: number | undefined
The time in milliseconds to wait before a long touch is performed.longPressDuration?: number;
/**
* The name of the parent (used for accepts function for increased specificity).
*/
ParentConfig<T>.name?: string | undefined
The name of the parent (used for accepts function for increased specificity).name?: string;
ParentConfig<T>.multiDrag?: boolean | undefined
multiDrag?: boolean;
/**
* If set to false, the library will not use the native drag and drop API.
*/
ParentConfig<T>.nativeDrag?: boolean | undefined
If set to false, the library will not use the native drag and drop API.nativeDrag?: boolean;
/**
* Function that is called when a sort operation is to be performed.
*/
ParentConfig<T>.performSort: ({ parent, draggedNodes, targetNodes, }: {
parent: ParentRecord<T>;
draggedNodes: Array<NodeRecord<T>>;
targetNodes: Array<NodeRecord<T>>;
}) => void
Function that is called when a sort operation is to be performed.performSort: ({
parent: ParentRecord<T>
parent,
draggedNodes: NodeRecord<T>[]
draggedNodes,
targetNodes: NodeRecord<T>[]
targetNodes,
}: {
parent: ParentRecord<T>
parent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
targetNodes: NodeRecord<T>[]
targetNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
}) => void;
/**
* Function that is called when a transfer operation is to be performed.
*/
ParentConfig<T>.performTransfer: ({ currentParent, targetParent, initialParent, draggedNodes, initialIndex, state, targetNodes, }: {
currentParent: ParentRecord<T>;
targetParent: ParentRecord<T>;
initialParent: ParentRecord<T>;
draggedNodes: Array<NodeRecord<T>>;
initialIndex: number;
state: BaseDragState<T> | DragState<T> | SynthDragState<T>;
targetNodes: Array<NodeRecord<T>>;
}) => void
Function that is called when a transfer operation is to be performed.performTransfer: ({
currentParent: ParentRecord<T>
currentParent,
targetParent: ParentRecord<T>
targetParent,
initialParent: ParentRecord<T>
initialParent,
draggedNodes: NodeRecord<T>[]
draggedNodes,
initialIndex: number
initialIndex,
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state,
targetNodes: NodeRecord<T>[]
targetNodes,
}: {
currentParent: ParentRecord<T>
currentParent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
targetParent: ParentRecord<T>
targetParent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
initialParent: ParentRecord<T>
initialParent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
initialIndex: number
initialIndex: number;
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state: type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T> | type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>;
targetNodes: NodeRecord<T>[]
targetNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
}) => void;
/**
* An array of functions to use for a given parent.
*/
ParentConfig<T>.plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins?: interface Array<T>
Array<type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin>;
/**
* Takes a given node and reapplies the drag classes.
*/
ParentConfig<T>.reapplyDragClasses: (node: Node, parentData: ParentData<T>) => void
Takes a given node and reapplies the drag classes.reapplyDragClasses: (node: Node
node: Node, parentData: ParentData<T>
parentData: interface ParentData<T>
The data assigned to a given parent in the `parents` weakmap.ParentData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Invoked when the remapping of a given parent's nodes is finished.
*/
ParentConfig<T>.remapFinished: (data: ParentData<T>) => void
Invoked when the remapping of a given parent's nodes is finished.remapFinished: (data: ParentData<T>
data: interface ParentData<T>
The data assigned to a given parent in the `parents` weakmap.ParentData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* The root element to use for the parent.
*/
ParentConfig<T>.root: Document | ShadowRoot
The root element to use for the parent.root: Document | ShadowRoot;
ParentConfig<T>.selectedClass?: string | undefined
selectedClass?: string;
/**
* Function that is called when a node is set up.
*/
ParentConfig<T>.setupNode: SetupNode
Function that is called when a node is set up.setupNode: type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode;
/**
* Called when the value of the parent is changed and the nodes are remapped.
*/
ParentConfig<T>.setupNodeRemap: SetupNode
Called when the value of the parent is changed and the nodes are remapped.setupNodeRemap: type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode;
/**
* Flag for whether or not to allow sorting within a given parent.
*/
ParentConfig<T>.sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable?: boolean;
/**
* The class to add to a parent when it is dragged over.
*/
ParentConfig<T>.synthDropZoneParentClass?: string | undefined
The class to add to a parent when it is dragged over.synthDropZoneParentClass?: string;
/**
* A function that returns the image to use for the drag operation. This is
* invoked for synth drag operations operations. The clonedNode is what will
* be set as the drag image, but this can be updated.
*/
ParentConfig<T>.synthDragImage?: ((node: NodeRecord<T>, parent: ParentRecord<T>, e: PointerEvent, draggedNodes: Array<NodeRecord<T>>) => {
dragImage: HTMLElement;
offsetX?: number;
offsetY?: number;
}) | undefined
A function that returns the image to use for the drag operation. This is
invoked for synth drag operations operations. The clonedNode is what will
be set as the drag image, but this can be updated.synthDragImage?: (
node: NodeRecord<T>
node: interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>,
parent: ParentRecord<T>
parent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
e: PointerEvent
e: PointerEvent,
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>
) => {
dragImage: HTMLElement
dragImage: HTMLElement;
offsetX?: number | undefined
offsetX?: number;
offsetY?: number | undefined
offsetY?: number;
};
/**
* Function that is called when a node is torn down.
*/
ParentConfig<T>.tearDownNode: TearDownNode
Function that is called when a node is torn down.tearDownNode: type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode;
/**
* Called when the value of the parent is changed and the nodes are remapped.
*/
ParentConfig<T>.tearDownNodeRemap: TearDownNode
Called when the value of the parent is changed and the nodes are remapped.tearDownNodeRemap: type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode;
/**
* Property to identify which group of tree descendants the current parent belongs to.
*/
/**
* The threshold for a drag to be considered a valid sort
* operation.
*/
ParentConfig<T>.threshold: {
horizontal: number;
vertical: number;
}
The threshold for a drag to be considered a valid sort
operation.threshold: {
horizontal: number
horizontal: number;
vertical: number
vertical: number;
};
/**
* The class to add to a node when it is being synthetically dragged.
*/
ParentConfig<T>.synthDraggingClass?: string | undefined
The class to add to a node when it is being synthetically dragged.synthDraggingClass?: string;
/**
* On synth drag start, this is applied to the dragged node(s) (not their
* representations being dragged).
*/
ParentConfig<T>.synthDragPlaceholderClass?: string | undefined
On synth drag start, this is applied to the dragged node(s) (not their
representations being dragged).synthDragPlaceholderClass?: string;
/**
* When hovering over a node, this class is applied to the node.
*/
ParentConfig<T>.synthDropZoneClass?: string | undefined
When hovering over a node, this class is applied to the node.synthDropZoneClass?: string;
/**
* When a node receives focus, this class is applied to the node.
*/
ParentConfig<T>.synthActiveDescendantClass?: string | undefined
When a node receives focus, this class is applied to the node.synthActiveDescendantClass?: string;
/**
* Config option to allow recursive copying of computed styles of dragged
* element to the cloned one that will be dragged (only for synthetic drag).
*/
ParentConfig<T>.deepCopyStyles?: boolean | undefined
Config option to allow recursive copying of computed styles of dragged
element to the cloned one that will be dragged (only for synthetic drag).deepCopyStyles?: boolean;
/**
* Callback function for when a sort operation is performed.
*/
ParentConfig<T>.onSort?: SortEvent | undefined
Callback function for when a sort operation is performed.onSort?: type SortEvent = <T>(data: SortEventData<T>) => void
SortEvent;
/**
* Callback function for when a transfer operation is performed.
*/
ParentConfig<T>.onTransfer?: TransferEvent | undefined
Callback function for when a transfer operation is performed.onTransfer?: type TransferEvent = <T>(data: TransferEventData<T>) => void
TransferEvent;
/**
* Fired when a drag is started, whether native drag or synthetic
*/
ParentConfig<T>.onDragstart?: DragstartEvent | undefined
Fired when a drag is started, whether native drag or syntheticonDragstart?: type DragstartEvent = <T>(data: DragstartEventData<T>) => void
DragstartEvent;
/**
* Fired when a drag is ended, whether native drag or synthetic
*/
ParentConfig<T>.onDragend?: DragendEvent | undefined
Fired when a drag is ended, whether native drag or syntheticonDragend?: type DragendEvent = <T>(data: DragendEventData<T>) => void
DragendEvent;
}
import type {
interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord,
type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState,
interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData,
interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData,
interface NodeEventData<T>
The data passed to the node event listener.NodeEventData,
type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin,
interface PointeroverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.PointeroverNodeEvent,
type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode,
interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData,
type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode,
interface PointeroverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.PointeroverParentEvent,
type SortEvent = <T>(data: SortEventData<T>) => void
SortEvent,
type TransferEvent = <T>(data: TransferEventData<T>) => void
TransferEvent,
type DragstartEvent = <T>(data: DragstartEventData<T>) => void
DragstartEvent,
type DragendEvent = <T>(data: DragendEventData<T>) => void
DragendEvent,
interface ParentDragEventData<T>
ParentDragEventData,
type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState,
type NativeDragEffects = "link" | "none" | "copy" | "move"
NativeDragEffects,
interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord,
interface DropSwapConfig<T>
DropSwapConfig,
interface InsertConfig<T>
InsertConfig,
interface ParentData<T>
The data assigned to a given parent in the `parents` weakmap.ParentData,
interface ParentKeydownEventData<T>
ParentKeydownEventData,
type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState,
} from "@formkit/drag-and-drop";
/**
* The configuration object for a given parent.
*/
export interface interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<function (type parameter) T in ParentConfig<T>
T> {
/**
* A function that returns whether a given parent accepts a given node.
*/
ParentConfig<T>.accepts?: ((targetParentData: ParentRecord<T>, initialParentData: ParentRecord<T>, currentParentData: ParentRecord<T>, state: BaseDragState<T>) => boolean) | undefined
A function that returns whether a given parent accepts a given node.accepts?: (
targetParentData: ParentRecord<T>
targetParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
initialParentData: ParentRecord<T>
initialParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
currentParentData: ParentRecord<T>
currentParentData: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T>
state: type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T>
) => boolean;
ParentConfig<T>.activeDescendantClass?: string | undefined
activeDescendantClass?: string;
/**
* The data transfer effect to use for the drag operation.
*/
ParentConfig<T>.dragEffectAllowed: NativeDragEffects
The data transfer effect to use for the drag operation.dragEffectAllowed: type NativeDragEffects = "link" | "none" | "copy" | "move"
NativeDragEffects;
/**
* The data transfer effect to use for the drag operation.
*/
ParentConfig<T>.dragDropEffect: NativeDragEffects
The data transfer effect to use for the drag operation.dragDropEffect: type NativeDragEffects = "link" | "none" | "copy" | "move"
NativeDragEffects;
/**
* A function that returns the image to use for the drag operation. This is
* invoked for native operations. The clonedNode is what will be set as the drag
* image, but this can be updated.
*/
ParentConfig<T>.dragImage?: ((data: NodeDragEventData<T>, draggedNodes: Array<NodeRecord<T>>) => HTMLElement) | undefined
A function that returns the image to use for the drag operation. This is
invoked for native operations. The clonedNode is what will be set as the drag
image, but this can be updated.dragImage?: (
data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>,
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>
) => HTMLElement;
/**
* A flag to disable dragability of all nodes in the parent.
*/
ParentConfig<T>.disabled?: boolean | undefined
A flag to disable dragability of all nodes in the parent.disabled?: boolean;
/**
* A selector for the drag handle. Will search at any depth within the
* draggable element.
*/
ParentConfig<T>.dragHandle?: string | undefined
A selector for the drag handle. Will search at any depth within the
draggable element.dragHandle?: string;
/**
* External drag handle
*/
ParentConfig<T>.externalDragHandle?: {
el: HTMLElement;
callback: () => HTMLElement;
} | undefined
External drag handleexternalDragHandle?: {
el: HTMLElement
el: HTMLElement;
callback: () => HTMLElement
callback: () => HTMLElement;
};
/**
* A function that returns whether a given node is draggable.
*/
ParentConfig<T>.draggable?: ((child: HTMLElement) => boolean) | undefined
A function that returns whether a given node is draggable.draggable?: (child: HTMLElement
child: HTMLElement) => boolean;
/**
* A function that returns whether a given value is draggable
*/
ParentConfig<T>.draggableValue?: ((values: T) => boolean) | undefined
A function that returns whether a given value is draggabledraggableValue?: (values: T
values: function (type parameter) T in ParentConfig<T>
T) => boolean;
ParentConfig<T>.draggedNodes: (pointerDown: {
parent: ParentRecord<T>;
node: NodeRecord<T>;
}) => Array<NodeRecord<T>>
draggedNodes: (pointerDown: {
parent: ParentRecord<T>;
node: NodeRecord<T>;
}
pointerDown: {
parent: ParentRecord<T>
parent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
node: NodeRecord<T>
node: interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>;
}) => interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
/**
* The class to add to a node when it is being dragged.
*/
ParentConfig<T>.draggingClass?: string | undefined
The class to add to a node when it is being dragged.draggingClass?: string;
/**
* Accepts array of "dragged nodes" and applies dragstart classes to them.
*/
ParentConfig<T>.dragstartClasses: (node: NodeRecord<T>, nodes: Array<NodeRecord<T>>, config: ParentConfig<T>, isSynthDrag?: boolean) => void
Accepts array of "dragged nodes" and applies dragstart classes to them.dragstartClasses: (
node: NodeRecord<T>
node: interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>,
nodes: NodeRecord<T>[]
nodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>,
config: ParentConfig<T>
config: interface ParentConfig<T>
The configuration object for a given parent.ParentConfig<function (type parameter) T in ParentConfig<T>
T>,
isSynthDrag: boolean | undefined
isSynthDrag?: boolean
) => void;
// When drag starts, this will be applied to the dragged node(s) (not their
// representations being dragged) on dragstart. This will remain on the nodes
// until the drag ends.
ParentConfig<T>.dragPlaceholderClass?: string | undefined
dragPlaceholderClass?: string;
/**
* The configuration object for the drop and swap plugin.
*/
ParentConfig<T>.dropSwapConfig?: DropSwapConfig<T> | undefined
The configuration object for the drop and swap plugin.dropSwapConfig?: interface DropSwapConfig<T>
DropSwapConfig<function (type parameter) T in ParentConfig<T>
T>;
/**
* The class to add to a node when the node is dragged over it.
*/
ParentConfig<T>.dropZoneClass?: string | undefined
The class to add to a node when the node is dragged over it.dropZoneClass?: string;
/**
* The class to add to a parent when it is dragged over.
*/
ParentConfig<T>.dropZoneParentClass?: string | undefined
The class to add to a parent when it is dragged over.dropZoneParentClass?: string;
/**
* A flag to indicate whether the parent itself is a dropZone.
*/
ParentConfig<T>.dropZone?: boolean | undefined
A flag to indicate whether the parent itself is a dropZone.dropZone?: boolean;
/**
* The group that the parent belongs to. This is used for allowing multiple
* parents to transfer nodes between each other.
*/
ParentConfig<T>.group?: string | undefined
The group that the parent belongs to. This is used for allowing multiple
parents to transfer nodes between each other.group?: string;
ParentConfig<T>.handleParentFocus: (data: ParentEventData<T>, state: BaseDragState<T>) => void
handleParentFocus: (
data: ParentEventData<T>
data: interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T>
state: type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
ParentConfig<T>.handleNodeKeydown: (data: NodeEventData<T>, state: DragState<T>) => void
handleNodeKeydown: (data: NodeEventData<T>
data: interface NodeEventData<T>
The data passed to the node event listener.NodeEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleParentKeydown: (data: ParentKeydownEventData<T>, state: DragState<T>) => void
handleParentKeydown: (
data: ParentKeydownEventData<T>
data: interface ParentKeydownEventData<T>
ParentKeydownEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when dragend or touchend event occurs.
*/
ParentConfig<T>.handleDragend: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when dragend or touchend event occurs.handleDragend: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when dragstart event occurs.
*/
ParentConfig<T>.handleDragstart: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when dragstart event occurs.handleDragstart: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleEnd: (state: DragState<T> | SynthDragState<T>) => void
handleEnd: (state: DragState<T> | SynthDragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleNodeDrop: (data: NodeDragEventData<T>, state: DragState<T>) => void
handleNodeDrop: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handleNodePointerup: (data: NodePointerEventData<T>, state: DragState<T>) => void
handleNodePointerup: (
data: NodePointerEventData<T>
data: interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
ParentConfig<T>.handleParentScroll: (data: ParentEventData<T>, state: DragState<T> | BaseDragState<T> | SynthDragState<T>) => void
handleParentScroll: (
data: ParentEventData<T>
data: interface ParentEventData<T>
The data passed to the parent event listener.ParentEventData<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a dragenter event is triggered on the node.
*/
ParentConfig<T>.handleNodeDragenter: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when a dragenter event is triggered on the node.handleNodeDragenter: (
data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Dragleave event on node
*/
ParentConfig<T>.handleNodeDragleave: (data: NodeDragEventData<T>, state: DragState<T>) => void
Dragleave event on nodehandleNodeDragleave: (
data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a dragover event is triggered on the parent.
*/
ParentConfig<T>.handleParentDragover: (data: ParentDragEventData<T>, state: DragState<T>) => void
Function that is called when a dragover event is triggered on the parent.handleParentDragover: (
data: ParentDragEventData<T>
data: interface ParentDragEventData<T>
ParentDragEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Drop event on parent
*/
ParentConfig<T>.handleParentDrop: (data: ParentDragEventData<T>, state: DragState<T>) => void
Drop event on parenthandleParentDrop: (data: ParentDragEventData<T>
data: interface ParentDragEventData<T>
ParentDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Function that is called when a dragover event is triggered on a node.
*/
ParentConfig<T>.handleNodeDragover: (data: NodeDragEventData<T>, state: DragState<T>) => void
Function that is called when a dragover event is triggered on a node.handleNodeDragover: (data: NodeDragEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T>, state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>) => void;
ParentConfig<T>.handlePointercancel: (data: NodeDragEventData<T> | NodePointerEventData<T>, state: DragState<T> | SynthDragState<T> | BaseDragState<T>) => void
handlePointercancel: (
data: NodeDragEventData<T> | NodePointerEventData<T>
data: interface NodeDragEventData<T>
The data passed to the node event listener when the event is a drag event.NodeDragEventData<function (type parameter) T in ParentConfig<T>
T> | interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData<function (type parameter) T in ParentConfig<T>
T>,
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T> | type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/*
* Function that is called when a pointerdown is triggered on node.
*/
ParentConfig<T>.handleNodePointerdown: (data: NodePointerEventData<T>, state: DragState<T>) => void
handleNodePointerdown: (
data: NodePointerEventData<T>
data: interface NodePointerEventData<T>
The data passed to the node event listener when the event is a pointer event (not a native drag event).NodePointerEventData<function (type parameter) T in ParentConfig<T>
T>,
state: DragState<T>
state: type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over a given node (similar to dragover).
*/
ParentConfig<T>.handleNodePointerover: (data: PointeroverNodeEvent<T>, state: SynthDragState<T>) => void
Function that is called when a node that is being moved by touchmove event
is over a given node (similar to dragover).handleNodePointerover: (
data: PointeroverNodeEvent<T>
data: interface PointeroverNodeEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
node.PointeroverNodeEvent<function (type parameter) T in ParentConfig<T>
T>,
state: SynthDragState<T>
state: type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Function that is called when a node that is being moved by touchmove event
* is over the parent (similar to dragover).
*/
ParentConfig<T>.handleParentPointerover: (e: PointeroverParentEvent<T>, state: SynthDragState<T>) => void
Function that is called when a node that is being moved by touchmove event
is over the parent (similar to dragover).handleParentPointerover: (
e: PointeroverParentEvent<T>
e: interface PointeroverParentEvent<T>
The payload of the custom event dispatched when a node is "touched" over a
parent.PointeroverParentEvent<function (type parameter) T in ParentConfig<T>
T>,
state: SynthDragState<T>
state: type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>
) => void;
/**
* Config option for insert plugin.
*/
ParentConfig<T>.insertConfig?: InsertConfig<T> | undefined
Config option for insert plugin.insertConfig?: interface InsertConfig<T>
InsertConfig<function (type parameter) T in ParentConfig<T>
T>;
/**
* A flag to indicate whether long touch is enabled.
*/
ParentConfig<T>.longPress?: boolean | undefined
A flag to indicate whether long touch is enabled.longPress?: boolean;
/**
* The class to add to a node when a long touch action is performed.
*/
ParentConfig<T>.longPressClass?: string | undefined
The class to add to a node when a long touch action is performed.longPressClass?: string;
/**
* The time in milliseconds to wait before a long touch is performed.
*/
ParentConfig<T>.longPressDuration?: number | undefined
The time in milliseconds to wait before a long touch is performed.longPressDuration?: number;
/**
* The name of the parent (used for accepts function for increased specificity).
*/
ParentConfig<T>.name?: string | undefined
The name of the parent (used for accepts function for increased specificity).name?: string;
ParentConfig<T>.multiDrag?: boolean | undefined
multiDrag?: boolean;
/**
* If set to false, the library will not use the native drag and drop API.
*/
ParentConfig<T>.nativeDrag?: boolean | undefined
If set to false, the library will not use the native drag and drop API.nativeDrag?: boolean;
/**
* Function that is called when a sort operation is to be performed.
*/
ParentConfig<T>.performSort: ({ parent, draggedNodes, targetNodes, }: {
parent: ParentRecord<T>;
draggedNodes: Array<NodeRecord<T>>;
targetNodes: Array<NodeRecord<T>>;
}) => void
Function that is called when a sort operation is to be performed.performSort: ({
parent: ParentRecord<T>
parent,
draggedNodes: NodeRecord<T>[]
draggedNodes,
targetNodes: NodeRecord<T>[]
targetNodes,
}: {
parent: ParentRecord<T>
parent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
targetNodes: NodeRecord<T>[]
targetNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
}) => void;
/**
* Function that is called when a transfer operation is to be performed.
*/
ParentConfig<T>.performTransfer: ({ currentParent, targetParent, initialParent, draggedNodes, initialIndex, state, targetNodes, }: {
currentParent: ParentRecord<T>;
targetParent: ParentRecord<T>;
initialParent: ParentRecord<T>;
draggedNodes: Array<NodeRecord<T>>;
initialIndex: number;
state: BaseDragState<T> | DragState<T> | SynthDragState<T>;
targetNodes: Array<NodeRecord<T>>;
}) => void
Function that is called when a transfer operation is to be performed.performTransfer: ({
currentParent: ParentRecord<T>
currentParent,
targetParent: ParentRecord<T>
targetParent,
initialParent: ParentRecord<T>
initialParent,
draggedNodes: NodeRecord<T>[]
draggedNodes,
initialIndex: number
initialIndex,
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state,
targetNodes: NodeRecord<T>[]
targetNodes,
}: {
currentParent: ParentRecord<T>
currentParent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
targetParent: ParentRecord<T>
targetParent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
initialParent: ParentRecord<T>
initialParent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>;
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
initialIndex: number
initialIndex: number;
state: BaseDragState<T> | DragState<T> | SynthDragState<T>
state: type BaseDragState<T> = {
activeState?: {
node: NodeRecord<T>;
parent: ParentRecord<T>;
};
affectedNodes: Array<NodeRecord<T>>;
currentTargetValue: T | undefined;
emit: (event: string, data: unknown) => void;
... 12 more ...;
rootUserSelect: string | undefined;
}
BaseDragState<function (type parameter) T in ParentConfig<T>
T> | type DragState<T> = DragStateProps<T> & BaseDragState<T>
DragState<function (type parameter) T in ParentConfig<T>
T> | type SynthDragState<T> = SynthDragStateProps & DragStateProps<T> & BaseDragState<T>
The state of the current drag. State is only created when a drag start
event is triggered.SynthDragState<function (type parameter) T in ParentConfig<T>
T>;
targetNodes: NodeRecord<T>[]
targetNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>;
}) => void;
/**
* An array of functions to use for a given parent.
*/
ParentConfig<T>.plugins?: DNDPlugin[] | undefined
An array of functions to use for a given parent.plugins?: interface Array<T>
Array<type DNDPlugin = (parent: HTMLElement) => DNDPluginData | undefined
DNDPlugin>;
/**
* Takes a given node and reapplies the drag classes.
*/
ParentConfig<T>.reapplyDragClasses: (node: Node, parentData: ParentData<T>) => void
Takes a given node and reapplies the drag classes.reapplyDragClasses: (node: Node
node: Node, parentData: ParentData<T>
parentData: interface ParentData<T>
The data assigned to a given parent in the `parents` weakmap.ParentData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* Invoked when the remapping of a given parent's nodes is finished.
*/
ParentConfig<T>.remapFinished: (data: ParentData<T>) => void
Invoked when the remapping of a given parent's nodes is finished.remapFinished: (data: ParentData<T>
data: interface ParentData<T>
The data assigned to a given parent in the `parents` weakmap.ParentData<function (type parameter) T in ParentConfig<T>
T>) => void;
/**
* The root element to use for the parent.
*/
ParentConfig<T>.root: Document | ShadowRoot
The root element to use for the parent.root: Document | ShadowRoot;
ParentConfig<T>.selectedClass?: string | undefined
selectedClass?: string;
/**
* Function that is called when a node is set up.
*/
ParentConfig<T>.setupNode: SetupNode
Function that is called when a node is set up.setupNode: type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode;
/**
* Called when the value of the parent is changed and the nodes are remapped.
*/
ParentConfig<T>.setupNodeRemap: SetupNode
Called when the value of the parent is changed and the nodes are remapped.setupNodeRemap: type SetupNode = <T>(data: SetupNodeData<T>) => void
SetupNode;
/**
* Flag for whether or not to allow sorting within a given parent.
*/
ParentConfig<T>.sortable?: boolean | undefined
Flag for whether or not to allow sorting within a given parent.sortable?: boolean;
/**
* The class to add to a parent when it is dragged over.
*/
ParentConfig<T>.synthDropZoneParentClass?: string | undefined
The class to add to a parent when it is dragged over.synthDropZoneParentClass?: string;
/**
* A function that returns the image to use for the drag operation. This is
* invoked for synth drag operations operations. The clonedNode is what will
* be set as the drag image, but this can be updated.
*/
ParentConfig<T>.synthDragImage?: ((node: NodeRecord<T>, parent: ParentRecord<T>, e: PointerEvent, draggedNodes: Array<NodeRecord<T>>) => {
dragImage: HTMLElement;
offsetX?: number;
offsetY?: number;
}) | undefined
A function that returns the image to use for the drag operation. This is
invoked for synth drag operations operations. The clonedNode is what will
be set as the drag image, but this can be updated.synthDragImage?: (
node: NodeRecord<T>
node: interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>,
parent: ParentRecord<T>
parent: interface ParentRecord<T>
The parent record, contains the el and the data in the `parents` weakmap.ParentRecord<function (type parameter) T in ParentConfig<T>
T>,
e: PointerEvent
e: PointerEvent,
draggedNodes: NodeRecord<T>[]
draggedNodes: interface Array<T>
Array<interface NodeRecord<T>
The node record, contains the el and the data in the `nodes` weakmap.NodeRecord<function (type parameter) T in ParentConfig<T>
T>>
) => {
dragImage: HTMLElement
dragImage: HTMLElement;
offsetX?: number | undefined
offsetX?: number;
offsetY?: number | undefined
offsetY?: number;
};
/**
* Function that is called when a node is torn down.
*/
ParentConfig<T>.tearDownNode: TearDownNode
Function that is called when a node is torn down.tearDownNode: type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode;
/**
* Called when the value of the parent is changed and the nodes are remapped.
*/
ParentConfig<T>.tearDownNodeRemap: TearDownNode
Called when the value of the parent is changed and the nodes are remapped.tearDownNodeRemap: type TearDownNode = <T>(data: TearDownNodeData<T>) => void
TearDownNode;
/**
* Property to identify which group of tree descendants the current parent belongs to.
*/
/**
* The threshold for a drag to be considered a valid sort
* operation.
*/
ParentConfig<T>.threshold: {
horizontal: number;
vertical: number;
}
The threshold for a drag to be considered a valid sort
operation.threshold: {
horizontal: number
horizontal: number;
vertical: number
vertical: number;
};
/**
* The class to add to a node when it is being synthetically dragged.
*/
ParentConfig<T>.synthDraggingClass?: string | undefined
The class to add to a node when it is being synthetically dragged.synthDraggingClass?: string;
/**
* On synth drag start, this is applied to the dragged node(s) (not their
* representations being dragged).
*/
ParentConfig<T>.synthDragPlaceholderClass?: string | undefined
On synth drag start, this is applied to the dragged node(s) (not their
representations being dragged).synthDragPlaceholderClass?: string;
/**
* When hovering over a node, this class is applied to the node.
*/
ParentConfig<T>.synthDropZoneClass?: string | undefined
When hovering over a node, this class is applied to the node.synthDropZoneClass?: string;
/**
* When a node receives focus, this class is applied to the node.
*/
ParentConfig<T>.synthActiveDescendantClass?: string | undefined
When a node receives focus, this class is applied to the node.synthActiveDescendantClass?: string;
/**
* Config option to allow recursive copying of computed styles of dragged
* element to the cloned one that will be dragged (only for synthetic drag).
*/
ParentConfig<T>.deepCopyStyles?: boolean | undefined
Config option to allow recursive copying of computed styles of dragged
element to the cloned one that will be dragged (only for synthetic drag).deepCopyStyles?: boolean;
/**
* Callback function for when a sort operation is performed.
*/
ParentConfig<T>.onSort?: SortEvent | undefined
Callback function for when a sort operation is performed.onSort?: type SortEvent = <T>(data: SortEventData<T>) => void
SortEvent;
/**
* Callback function for when a transfer operation is performed.
*/
ParentConfig<T>.onTransfer?: TransferEvent | undefined
Callback function for when a transfer operation is performed.onTransfer?: type TransferEvent = <T>(data: TransferEventData<T>) => void
TransferEvent;
/**
* Fired when a drag is started, whether native drag or synthetic
*/
ParentConfig<T>.onDragstart?: DragstartEvent | undefined
Fired when a drag is started, whether native drag or syntheticonDragstart?: type DragstartEvent = <T>(data: DragstartEventData<T>) => void
DragstartEvent;
/**
* Fired when a drag is ended, whether native drag or synthetic
*/
ParentConfig<T>.onDragend?: DragendEvent | undefined
Fired when a drag is ended, whether native drag or syntheticonDragend?: type DragendEvent = <T>(data: DragendEventData<T>) => void
DragendEvent;
}
Accessibility
By adding appropriate aria-label
attributes to both the parent container and the draggable items, you can make your lists accessible to screen readers.
Support Us
FormKit Drag and Drop is made with love by the FormKit team — the creators of open-source projects such as:
- FormKit - The open-source form framework for Vue.
- AutoAnimate - Add motion to your apps with a single line of code.
- Tempo - The easiest way to work with dates in JavaScript.
- ArrowJS - Reactivity without the Framework.
If you find our projects useful and would like to support their ongoing development, please consider sponsoring us on GitHub!